
Gnome::Gio::ListStore
Description
Gnome::Gio::ListStore is a simple implementation of Gnome::Gio::ListStore that stores all items in memory.
It provides insertions, deletions, and lookups in logarithmic time with a fast path for the common case of iterating the list linearly.
Uml Diagram
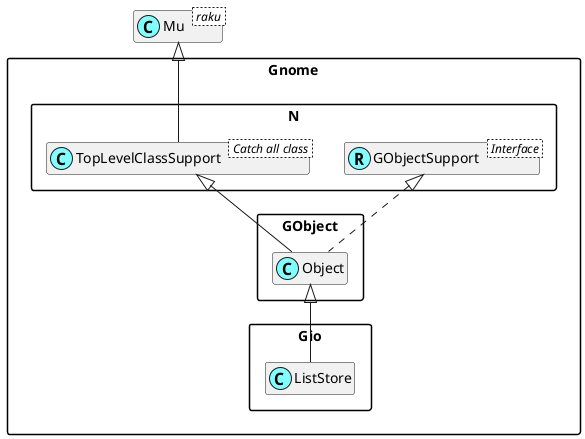
Class initialization
new
:native-object
Create an object using a native object from an object of the same type found elsewhere. See also Gnome::N::TopLevelSupportClass.
multi method new ( N-Object() :$native-object! )
new-liststore
Creates a new Gnome::Gio::ListStore with items of type $item-type
. $item-type
must be a subclass of Gnome::GObject::Object.
method new-liststore ( GType $item-type --> Gnome::Gio::ListStore \)
$item-type; the Gnome::GObject::ListStore of items in the list.
Methods
append
Appends $item
to $store
. $item
must be of type Gnome::Gio::ListStore:item-type.
This function takes a ref on $item
.
Use .splice()
to append multiple items at the same time efficiently.
method append ( gpointer $item )
$item; the new item.
find
Looks up the given $item
in the list store by looping over the items until the first occurrence of $item
. If $item
was not found, then $position
will not be set, and this method will return False
.
If you need to compare the two items with a custom comparison function, use .find-with-equal-func()
with a custom Gnome::Glib::T-types instead.
method find ( gpointer $item, Array[Int] $position --> Bool )
$item; an item.
$position; (transfer ownership: full) the first position of
$item
, if it was found..
Return value; Whether $store
contains $item
. If it was found, $position
will be set to the position where $item
occurred for the first time..
find-with-equal-func
Looks up the given $item
in the list store by looping over the items and comparing them with $equal-func
until the first occurrence of $item
which matches. If $item
was not found, then $position
will not be set, and this method will return False
. $item
is always passed as second parameter to $equal-func
.
Since GLib 2.76 it is possible to pass undefined for $item
.
method find-with-equal-func ( gpointer $item, GEqualFunc &equal-func, Array[Int] $position --> Bool )
$item; an item.
GEqualFunc &equal-func; A custom equality check function. The function must be specified with the following signature;
:( gpointer $a, gpointer $b )
.$position; (transfer ownership: full) the first position of
$item
, if it was found..
Return value; Whether $store
contains $item
. If it was found, $position
will be set to the position where $item
occurred for the first time..
find-with-equal-func-full
Like .find-with-equal-func()
but with an additional $user-data
that is passed to $equal-func
. $item
is always passed as second parameter to $equal-func
.
Since GLib 2.76 it is possible to pass undefined for $item
.
method find-with-equal-func-full ( gpointer $item, GEqualFuncFull &equal-func, gpointer $user-data, Array[Int] $position --> Bool )
$item; an item.
GEqualFuncFull &equal-func; A custom equality check function. The function must be specified with the following signature;
:( gpointer $a, gpointer $b, gpointer $user-data )
.$user-data; user data for
$equal-func
.$position; (transfer ownership: full) the first position of
$item
, if it was found..
Return value; Whether $store
contains $item
. If it was found, $position
will be set to the position where $item
occurred for the first time..
insert
Inserts $item
into $store
at $position
. $item
must be of type Gnome::Gio::ListStore:item-type or derived from it. $position
must be smaller than the length of the list, or equal to it to append.
This function takes a ref on $item
.
Use .splice()
to insert multiple items at the same time efficiently.
method insert ( UInt() $position, gpointer $item )
$position; the position at which to insert the new item.
$item; the new item.
insert-sorted
Inserts $item
into $store
at a position to be determined by the $compare-func
.
The list must already be sorted before calling this function or the result is undefined. Usually you would approach this by only ever inserting items by way of this function.
This function takes a ref on $item
.
method insert-sorted ( gpointer $item, GCompareDataFunc &compare-func, gpointer $user-data --> UInt )
$item; the new item.
GCompareDataFunc &compare-func; pairwise comparison function for sorting. The function must be specified with the following signature;
:( gpointer $a, gpointer $b, gpointer $user-data )
.$user-data; user data for
$compare-func
.
Return value; the position at which $item
was inserted.
remove
Removes the item from $store
that is at $position
. $position
must be smaller than the current length of the list.
Use .splice()
to remove multiple items at the same time efficiently.
method remove ( UInt() $position )
$position; the position of the item that is to be removed.
remove-all
Removes all items from $store
.
method remove-all ( )
sort
Sort the items in $store
according to $compare-func
.
method sort ( GCompareDataFunc &compare-func, gpointer $user-data )
GCompareDataFunc &compare-func; pairwise comparison function for sorting. The function must be specified with the following signature;
:( gpointer $a, gpointer $b, gpointer $user-data )
.$user-data; user data for
$compare-func
.
splice
Changes $store
by removing $n-removals
items and adding $n-additions
items to it. $additions
must contain $n-additions
items of type Gnome::Gio::ListStore:item-type. undefined is not permitted.
This function is more efficient than .insert()
and .remove()
, because it only emits items-changed defined in Gnome::Gio::ListStore
once for the change.
This function takes a ref on each item in $additions
.
The parameters $position
and $n-removals
must be correct (ie: $position
+ $n-removals
must be less than or equal to the length of the list at the time this function is called).
method splice ( UInt() $position, UInt() $n-removals, Array $additions, UInt() $n-additions )
$position; the position at which to make the change.
$n-removals; the number of items to remove.
$additions; the items to add.
$n-additions; the number of items to add.