
Gnome::Gio::SimpleAsyncResult
Description
As of GLib 2.46, Gnome::Gio::SimpleAsyncResult is deprecated in favor of Gnome::Gio::SimpleAsyncResult, which provides a simpler API.
Gnome::Gio::SimpleAsyncResult implements Gnome::Gio::SimpleAsyncResult.
Gnome::Gio::SimpleAsyncResult handles [type $Gio
.AsyncReadyCallback]s, error reporting, operation cancellation and the final state of an operation, completely transparent to the application. Results can be returned as a pointer e.g. for functions that return data that is collected asynchronously, a boolean value for checking the success or failure of an operation, or a gssize for operations which return the number of bytes modified by the operation; all of the simple return cases are covered.
Most of the time, an application will not need to know of the details of this API; it is handled transparently, and any necessary operations are handled by Gnome::Gio::SimpleAsyncResult’s interface. However, if implementing a new GIO module, for writing language bindings, or for complex applications that need better control of how asynchronous operations are completed, it is important to understand this functionality.
Gnome::Gio::SimpleAsyncResults are tagged with the calling function to ensure that asynchronous functions and their finishing functions are used together correctly.
To create a new Gnome::Gio::SimpleAsyncResult, call .newsimpleasyncresult()
. If the result needs to be created for a Gnome::Glib::N-Error, use .new-from-error()
or .new-take-error()
. If a Gnome::Glib::N-Error is not available (e.g. the asynchronous operation doesn’t take a Gnome::Glib::N-Error argument), but the result still needs to be created for an error condition, use .new-error()
(or .set-error-va()
if your application or binding requires passing a variable argument list directly), and the error can then be propagated through the use of .propagate-error()
.
An asynchronous operation can be made to ignore a cancellation event by calling .set-handle-cancellation()
with a Gnome::Gio::SimpleAsyncResult for the operation and False
. This is useful for operations that are dangerous to cancel, such as close (which would cause a leak if cancelled before being run).
Gnome::Gio::SimpleAsyncResult can integrate into GLib’s event loop, [type $GLib
.MainLoop], or it can use [type $GLib
.Thread]s. .complete()
will finish an I/O task directly from the point where it is called. .complete-in-idle()
will finish it from an idle handler in the thread-default main context (see .push-thread-default()
in class Gnome::Gio::SimpleAsyncResult
) where the Gnome::Gio::SimpleAsyncResult was created. .run-in-thread()
will run the job in a separate thread and then use .complete-in-idle()
to deliver the result.
To set the results of an asynchronous function, .set-op-res-gpointer()
, .set-op-res-gboolean()
, and .set-op-res-gssize()
are provided, setting the operation's result to a gpointer, gboolean, or gssize, respectively.
Likewise, to get the result of an asynchronous function, .get-op-res-gpointer()
, .get-op-res-gboolean()
, and .get-op-res-gssize()
are provided, getting the operation’s result as a gpointer, gboolean, and gssize, respectively.
For the details of the requirements implementations must respect, see Gnome::Gio::SimpleAsyncResult. A typical implementation of an asynchronous operation using Gnome::Gio::SimpleAsyncResult looks something like this:
Uml Diagram
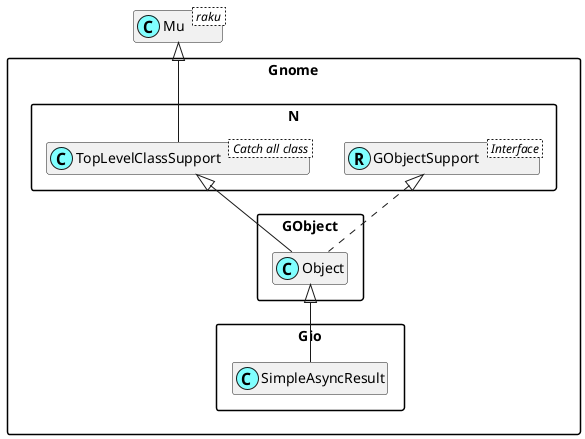
Class initialization
new
:native-object
Create an object using a native object from an object of the same type found elsewhere. See also Gnome::N::TopLevelSupportClass.
multi method new ( N-Object() :$native-object! )
new-simpleasyncresult
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Creates a Gnome::Gio::SimpleAsyncResult.
The common convention is to create the Gnome::Gio::SimpleAsyncResult in the function that starts the asynchronous operation and use that same function as the $source-tag
.
If your operation supports cancellation with Gnome::Gio::Cancellable (which it probably should) then you should provide the user's cancellable to .set-check-cancellable()
immediately after this function returns.
method new-simpleasyncresult ( N-Object() $source-object, GAsyncReadyCallback &callback, gpointer $user-data, gpointer $source-tag --> Gnome::Gio::SimpleAsyncResult \)
$source-object; a Gnome::GObject::Object, or undefined..
GAsyncReadyCallback &callback; a Gnome::Gio::T-iotypes.. The function must be specified with the following signature;
:( N-Object $source-object, N-Object $res, gpointer $data )
.$user-data; user data passed to
$callback
..$source-tag; the asynchronous function..
new-error This function is not yet available
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Creates a new Gnome::Gio::SimpleAsyncResult with a set error.
method new-error ( N-Object() $source-object, GAsyncReadyCallback &callback, gpointer $user-data, UInt $domain, Int() $code, Str $format, … --> Gnome::Gio::SimpleAsyncResult \)
$source-object; a Gnome::GObject::Object, or undefined..
GAsyncReadyCallback &callback; a Gnome::Gio::T-iotypes.. The function must be specified with the following signature;
:( N-Object $source-object, N-Object $res, gpointer $data )
.$user-data; user data passed to
$callback
..$domain; a Gnome::Glib::SimpleAsyncResult..
$code; an error code..
$format; a string with format characters..
…; …. Note that each argument must be specified as a type followed by its value!
new-from-error
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Creates a Gnome::Gio::SimpleAsyncResult from an error condition.
method new-from-error ( N-Object() $source-object, GAsyncReadyCallback &callback, gpointer $user-data, N-Object $error --> Gnome::Gio::SimpleAsyncResult \)
$source-object; a Gnome::GObject::Object, or undefined..
GAsyncReadyCallback &callback; a Gnome::Gio::T-iotypes.. The function must be specified with the following signature;
:( N-Object $source-object, N-Object $res, gpointer $data )
.$user-data; user data passed to
$callback
..$error; a Gnome::Glib::N-Error
new-take-error
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Creates a Gnome::Gio::SimpleAsyncResult from an error condition, and takes over the caller's ownership of $error
, so the caller does not need to free it anymore.
method new-take-error ( N-Object() $source-object, GAsyncReadyCallback &callback, gpointer $user-data, N-Object $error --> Gnome::Gio::SimpleAsyncResult \)
$source-object; a Gnome::GObject::Object, or undefined.
GAsyncReadyCallback &callback; a Gnome::Gio::T-iotypes.. The function must be specified with the following signature;
:( N-Object $source-object, N-Object $res, gpointer $data )
.$user-data; user data passed to
$callback
..$error; a Gnome::Glib::N-Error
Methods
complete
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Completes an asynchronous I/O job immediately. Must be called in the thread where the asynchronous result was to be delivered, as it invokes the callback directly. If you are in a different thread use .complete-in-idle()
.
Calling this function takes a reference to $simple
for as long as is needed to complete the call.
method complete ( )
complete-in-idle
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Completes an asynchronous function in an idle handler in the thread-default main context of the thread that $simple
was initially created in (and re-pushes that context around the invocation of the callback).
Calling this function takes a reference to $simple
for as long as is needed to complete the call.
method complete-in-idle ( )
get-op-res-gboolean
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Gets the operation result boolean from within the asynchronous result.
method get-op-res-gboolean (--> Bool )
Return value; True
if the operation's result was True
, False
if the operation's result was False
..
get-op-res-gpointer
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Gets a pointer result as returned by the asynchronous function.
method get-op-res-gpointer (--> gpointer )
Return value; a pointer from the result..
get-op-res-gssize
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Gets a gssize from the asynchronous result.
method get-op-res-gssize (--> Int )
Return value; a gssize returned from the asynchronous function..
get-source-tag
Note: The native version of this routine is deprecated in gio-lib() since version 2.46.
Gets the source tag for the Gnome::Gio::SimpleAsyncResult.
method get-source-tag (--> gpointer )
Return value; a #gpointer to the source object for the Gnome::Gio::SimpleAsyncResult..
propagate-error
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Propagates an error from within the simple asynchronous result to a given destination.
If the Gnome::Gio::Cancellable given to a prior call to .set-check-cancellable()
is cancelled then this function will return True
with $dest
set appropriately.
method propagate-error ( CArray[N-Error] $err --> Bool )
$err; Error object. When defined, an error can be returned when there is one. Use
Pointer
when you want to ignore the error. .
Return value; True
if the error was propagated to $dest
. False
otherwise..
run-in-thread
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Runs the asynchronous job in a separate thread and then calls .complete-in-idle()
on $simple
to return the result to the appropriate main loop.
Calling this function takes a reference to $simple
for as long as is needed to run the job and report its completion.
method run-in-thread ( GSimpleAsyncThreadFunc &func, Int() $io-priority, N-Object() $cancellable )
GSimpleAsyncThreadFunc &func; a Gnome::Gio::T-iotypes.. The function must be specified with the following signature;
:( N-Object $res, N-Object $object, N-Object $cancellable )
.$io-priority; the io priority of the request..
$cancellable; optional Gnome::Gio::Cancellable object, undefined to ignore..
set-check-cancellable
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Sets a Gnome::Gio::Cancellable to check before dispatching results.
This function has one very specific purpose: the provided cancellable is checked at the time of .propagate-error()
If it is cancelled, these functions will return an "Operation was cancelled" error (G_IO_ERROR_CANCELLED
).
Implementors of cancellable asynchronous functions should use this in order to provide a guarantee to their callers that cancelling an async operation will reliably result in an error being returned for that operation (even if a positive result for the operation has already been sent as an idle to the main context to be dispatched).
The checking described above is done regardless of any call to the unrelated .set-handle-cancellation()
function.
method set-check-cancellable ( N-Object() $check-cancellable )
$check-cancellable; a Gnome::Gio::Cancellable to check, or undefined to unset.
set-error This function is not yet available
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Sets an error within the asynchronous result without a Gnome::Glib::N-Error.
method set-error ( UInt $domain, Int() $code, Str $format, … )
$domain; a Gnome::Glib::SimpleAsyncResult (usually
G_IO_ERROR
)..$code; an error code..
$format; a formatted error reporting string..
…; …. Note that each argument must be specified as a type followed by its value!
set-error-va This function is not yet available
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Sets an error within the asynchronous result without a Gnome::Glib::N-Error. Unless writing a binding, see .set-error()
.
method set-error-va ( UInt $domain, Int() $code, Str $format, … )
$domain; a Gnome::Glib::SimpleAsyncResult (usually
G_IO_ERROR
)..$code; an error code..
$format; a formatted error reporting string..
args; va_list of arguments.. Note that each argument must be specified as a type followed by its value!
set-from-error
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Sets the result from a Gnome::Glib::N-Error.
method set-from-error ( N-Object $error )
$error; Gnome::Glib::N-Error.
set-handle-cancellation
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Sets whether to handle cancellation within the asynchronous operation.
This function has nothing to do with .set-check-cancellable()
. It only refers to the Gnome::Gio::Cancellable passed to .run-in-thread()
.
method set-handle-cancellation ( Bool() $handle-cancellation )
$handle-cancellation; a #gboolean..
set-op-res-gboolean
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Sets the operation result to a boolean within the asynchronous result.
method set-op-res-gboolean ( Bool() $op-res )
$op-res; a #gboolean..
set-op-res-gpointer
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Sets the operation result within the asynchronous result to a pointer.
method set-op-res-gpointer ( gpointer $op-res, GDestroyNotify &destroy-op-res )
$op-res; a pointer result from an asynchronous function..
GDestroyNotify &destroy-op-res; a Gnome::Glib::T-types function.. The function must be specified with the following signature;
:( gpointer $data )
.
set-op-res-gssize
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Sets the operation result within the asynchronous result to the given $op-res
.
method set-op-res-gssize ( Int() $op-res )
$op-res; a #gssize..
take-error
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Sets the result from $error
, and takes over the caller's ownership of $error
, so the caller does not need to free it any more.
method take-error ( N-Object $error )
$error; a Gnome::Glib::N-Error
Functions
is-valid
Note: The native version of this routine is deprecated in gio-lib() since version 2.46
Ensures that the data passed to the _finish function of an async operation is consistent. Three checks are performed.
First, $result
is checked to ensure that it is really a Gnome::Gio::SimpleAsyncResult. Second, $source
is checked to ensure that it matches the source object of $result
. Third, $source-tag
is checked to ensure that it is equal to the $source-tag
argument given to .new-simpleasyncresult()
(which, by convention, is a pointer to the _async function corresponding to the _finish function from which this function is called). (Alternatively, if either $source-tag
or $result
's source tag is undefined, then the source tag check is skipped.)
method is-valid ( N-Object() $result, N-Object() $source, gpointer $source-tag --> Bool )
$result; the Gnome::Gio::R-AsyncResult passed to the _finish function..
$source; the Gnome::GObject::Object passed to the _finish function..
$source-tag; the asynchronous function..
Return value; #TRUE if all checks passed or #FALSE if any failed..