
Gnome::Gtk4::CellRenderer
Description
An object for rendering a single cell
The Gnome::Gtk4::CellRenderer is a base class of a set of objects used for rendering a cell to a Cairo::cairo_t. These objects are used primarily by the Gnome::Gtk4::TreeView widget, though they aren’t tied to them in any specific way. It is worth noting that Gnome::Gtk4::CellRenderer is not a Gnome::Gtk4::Widget and cannot be treated as such.
The primary use of a Gnome::Gtk4::CellRenderer is for drawing a certain graphical elements on a Cairo::cairo_t. Typically, one cell renderer is used to draw many cells on the screen. To this extent, it isn’t expected that a CellRenderer keep any permanent state around. Instead, any state is set just prior to use using Gnome::GObject::Objects property system. Then, the cell is measured using .get-preferred-size()
. Finally, the cell is rendered in the correct location using .snapshot()
.
There are a number of rules that must be followed when writing a new Gnome::Gtk4::CellRenderer. First and foremost, it’s important that a certain set of properties will always yield a cell renderer of the same size, barring a style change. The Gnome::Gtk4::CellRenderer also has a number of generic properties that are expected to be honored by all children.
Beyond merely rendering a cell, cell renderers can optionally provide active user interface elements. A cell renderer can be “activatable” like Gnome::Gtk4::CellRendererToggle, which toggles when it gets activated by a mouse click, or it can be “editable” like Gnome::Gtk4::CellRendererText, which allows the user to edit the text using a widget implementing the Gnome::Gtk4::R-CellEditable interface, e.g. Gnome::Gtk4::Entry. To make a cell renderer activatable or editable, you have to implement the Gnome::Gtk4::CellRendererClass.activate or Gnome::Gtk4::CellRendererClass.start_editing virtual functions, respectively.
Many properties of Gnome::Gtk4::CellRenderer and its subclasses have a corresponding “set” property, e.g. “cell-background-set” corresponds to “cell-background”. These “set” properties reflect whether a property has been set or not. You should not set them independently.
Uml Diagram
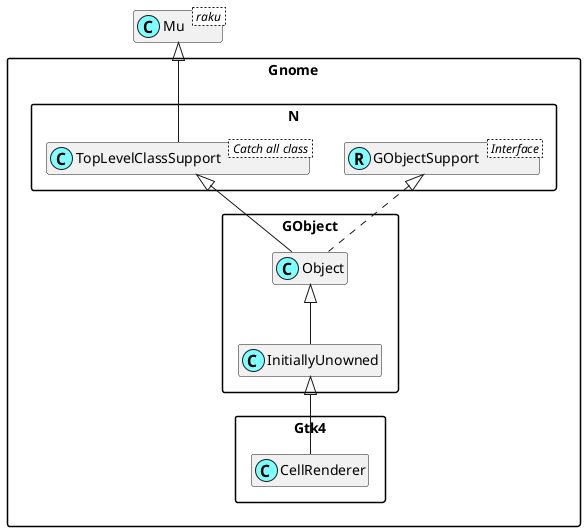
Class initialization
Note: The native version of this class is deprecated in gtk4-lib() since version 4.10
new
:native-object
Create an object using a native object from an object of the same type found elsewhere. See also Gnome::N::TopLevelSupportClass.
multi method new ( N-Object() :$native-object! )
Methods
activate
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Passes an activate event to the cell renderer for possible processing. Some cell renderers may use events; for example, Gnome::Gtk4::CellRendererToggle toggles when it gets a mouse click.
method activate ( N-Object() $event, N-Object() $widget, Str $path, N-Object $background-area, N-Object $cell-area, UInt $flags --> Bool )
$event; a Gnome::Gdk4::Event.
$widget; widget that received the event.
$path; widget-dependent string representation of the event location; e.g. for Gnome::Gtk4::TreeView, a string representation of Gnome::Gtk4::N-TreePath.
$background-area; background area as passed to
.render()
$cell-area; cell area as passed to
.render()
$flags; render flags.
Return value; True
if the event was consumed/handled.
get-aligned-area
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Gets the aligned area used by $cell
inside $cell-area
. Used for finding the appropriate edit and focus rectangle.
method get-aligned-area ( N-Object() $widget, UInt $flags, N-Object $cell-area, N-Object $aligned-area )
$widget; the Gnome::Gtk4::Widget this cell will be rendering to.
$flags; render flags.
$cell-area; cell area which would be passed to
.render()
$aligned-area; the return location for the space inside
$cell-area
that would actually be used to render.
get-alignment
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Fills in $xalign
and $yalign
with the appropriate values of $cell
.
method get-alignment ( Num() $xalign, Num() $yalign )
$xalign; (transfer ownership: full) location to fill in with the x alignment of the cell.
$yalign; (transfer ownership: full) location to fill in with the y alignment of the cell.
get-fixed-size
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Fills in $width
and $height
with the appropriate size of $cell
.
method get-fixed-size ( Array[Int] $width, Array[Int] $height )
$width; (transfer ownership: full) location to fill in with the fixed width of the cell.
$height; (transfer ownership: full) location to fill in with the fixed height of the cell.
get-is-expanded
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Checks whether the given Gnome::Gtk4::CellRenderer is expanded.
method get-is-expanded (--> Bool )
Return value; True
if the cell renderer is expanded.
get-is-expander
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Checks whether the given Gnome::Gtk4::CellRenderer is an expander.
method get-is-expander (--> Bool )
Return value; True
if $cell
is an expander, and False
otherwise.
get-padding
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Fills in $xpad
and $ypad
with the appropriate values of $cell
.
method get-padding ( Array[Int] $xpad, Array[Int] $ypad )
$xpad; (transfer ownership: full) location to fill in with the x padding of the cell.
$ypad; (transfer ownership: full) location to fill in with the y padding of the cell.
get-preferred-height
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Retrieves a renderer’s natural size when rendered to $widget
.
method get-preferred-height ( N-Object() $widget, Array[Int] $minimum-size, Array[Int] $natural-size )
$widget; the Gnome::Gtk4::Widget this cell will be rendering to.
$minimum-size; (transfer ownership: full) location to store the minimum size.
$natural-size; (transfer ownership: full) location to store the natural size.
get-preferred-height-for-width
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Retrieves a cell renderers’s minimum and natural height if it were rendered to $widget
with the specified $width
.
method get-preferred-height-for-width ( N-Object() $widget, Int() $width, Array[Int] $minimum-height, Array[Int] $natural-height )
$widget; the Gnome::Gtk4::Widget this cell will be rendering to.
$width; the size which is available for allocation.
$minimum-height; (transfer ownership: full) location for storing the minimum size.
$natural-height; (transfer ownership: full) location for storing the preferred size.
get-preferred-size
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Retrieves the minimum and natural size of a cell taking into account the widget’s preference for height-for-width management.
method get-preferred-size ( N-Object() $widget, N-Object $minimum-size, N-Object $natural-size )
$widget; the Gnome::Gtk4::Widget this cell will be rendering to.
$minimum-size; location for storing the minimum size
$natural-size; location for storing the natural size
get-preferred-width
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Retrieves a renderer’s natural size when rendered to $widget
.
method get-preferred-width ( N-Object() $widget, Array[Int] $minimum-size, Array[Int] $natural-size )
$widget; the Gnome::Gtk4::Widget this cell will be rendering to.
$minimum-size; (transfer ownership: full) location to store the minimum size.
$natural-size; (transfer ownership: full) location to store the natural size.
get-preferred-width-for-height
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Retrieves a cell renderers’s minimum and natural width if it were rendered to $widget
with the specified $height
.
method get-preferred-width-for-height ( N-Object() $widget, Int() $height, Array[Int] $minimum-width, Array[Int] $natural-width )
$widget; the Gnome::Gtk4::Widget this cell will be rendering to.
$height; the size which is available for allocation.
$minimum-width; (transfer ownership: full) location for storing the minimum size.
$natural-width; (transfer ownership: full) location for storing the preferred size.
get-request-mode
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Gets whether the cell renderer prefers a height-for-width layout or a width-for-height layout.
method get-request-mode (--> GtkSizeRequestMode )
Return value; The enumeration GtkSizeRequestMode defined in Gnome::Gtk4::T-enums
preferred by this renderer..
get-sensitive
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Returns the cell renderer’s sensitivity.
method get-sensitive (--> Bool )
Return value; True
if the cell renderer is sensitive.
get-state
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Translates the cell renderer state to bit field GtkStateFlags defined in Gnome::Gtk4::T-enums
, based on the cell renderer and widget sensitivity, and the given Gnome::Gtk4::CellRendererState.
method get-state ( N-Object() $widget, UInt $cell-state --> UInt )
$widget; a Gnome::Gtk4::Widget.
$cell-state; cell renderer state.
Return value; the widget state flags applying to $cell
.
get-visible
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Returns the cell renderer’s visibility.
method get-visible (--> Bool )
Return value; True
if the cell renderer is visible.
is-activatable
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Checks whether the cell renderer can do something when activated.
method is-activatable (--> Bool )
Return value; True
if the cell renderer can do anything when activated.
set-alignment
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Sets the renderer’s alignment within its available space.
method set-alignment ( Num() $xalign, Num() $yalign )
$xalign; the x alignment of the cell renderer.
$yalign; the y alignment of the cell renderer.
set-fixed-size
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Sets the renderer size to be explicit, independent of the properties set.
method set-fixed-size ( Int() $width, Int() $height )
$width; the width of the cell renderer, or -1.
$height; the height of the cell renderer, or -1.
set-is-expanded
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Sets whether the given Gnome::Gtk4::CellRenderer is expanded.
method set-is-expanded ( Bool() $is-expanded )
$is-expanded; whether
$cell
should be expanded.
set-is-expander
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Sets whether the given Gnome::Gtk4::CellRenderer is an expander.
method set-is-expander ( Bool() $is-expander )
$is-expander; whether
$cell
is an expander.
set-padding
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Sets the renderer’s padding.
method set-padding ( Int() $xpad, Int() $ypad )
$xpad; the x padding of the cell renderer.
$ypad; the y padding of the cell renderer.
set-sensitive
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Sets the cell renderer’s sensitivity.
method set-sensitive ( Bool() $sensitive )
$sensitive; the sensitivity of the cell.
set-visible
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Sets the cell renderer’s visibility.
method set-visible ( Bool() $visible )
$visible; the visibility of the cell.
snapshot
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Invokes the virtual render function of the Gnome::Gtk4::CellRenderer. The three passed-in rectangles are areas in $cr
. Most renderers will draw within $cell-area
; the xalign, yalign, xpad, and ypad fields of the Gnome::Gtk4::CellRenderer should be honored with respect to $cell-area
. $background-area
includes the blank space around the cell, and also the area containing the tree expander; so the $background-area
rectangles for all cells tile to cover the entire $window
.
method snapshot ( N-Object() $snapshot, N-Object() $widget, N-Object $background-area, N-Object $cell-area, UInt $flags )
$snapshot; a Gnome::Gtk4::Snapshot to draw to.
$widget; the widget owning
$window
.$background-area; entire cell area (including tree expanders and maybe padding on the sides)
$cell-area; area normally rendered by a cell renderer
$flags; flags that affect rendering.
start-editing
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Starts editing the contents of this $cell
, through a new Gnome::Gtk4::R-CellEditable widget created by the Gnome::Gtk4::CellRendererClass.start_editing virtual function.
method start-editing ( N-Object() $event, N-Object() $widget, Str $path, N-Object $background-area, N-Object $cell-area, UInt $flags --> N-Object )
$event; a Gnome::Gdk4::Event.
$widget; widget that received the event.
$path; widget-dependent string representation of the event location; e.g. for Gnome::Gtk4::TreeView, a string representation of Gnome::Gtk4::N-TreePath.
$background-area; background area as passed to
.render()
$cell-area; cell area as passed to
.render()
$flags; render flags.
Return value; A new Gnome::Gtk4::R-CellEditable for editing this $cell
, or undefined if editing is not possible.
stop-editing
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Informs the cell renderer that the editing is stopped. If $canceled
is True
, the cell renderer will emit the Gnome::Gtk4::CellRenderer::editing-canceled signal.
This function should be called by cell renderer implementations in response to the Gnome::Gtk4::R-CellEditable::editing-done` signal of Gnome::Gtk4::R-CellEditable.
method stop-editing ( Bool() $canceled )
$canceled;
True
if the editing has been canceled.
Signals
editing-canceled
This signal gets emitted when the user cancels the process of editing a cell. For example, an editable cell renderer could be written to cancel editing when the user presses Escape.
See also: .stop-editing()
.
method handler ( Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::CellRenderer :$_widget, *C<user>-options )
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::CellRenderer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
editing-started
This signal gets emitted when a cell starts to be edited. The intended use of this signal is to do special setup on $editable
, e.g. adding a Gnome::Gtk4::EntryCompletion or setting up additional columns in a Gnome::Gtk4::ComboBox.
See gtk_cell_editable_start_editing() for information on the lifecycle of the $editable
and a way to do setup that doesn’t depend on the $renderer
.
Note that GTK doesn't guarantee that cell renderers will continue to use the same kind of widget for editing in future releases, therefore you should check the type of $editable
before doing any specific setup, as in the following example:
method handler ( N-Object $editable, Str $path, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::CellRenderer :$_widget, *C<user>-options )
$editable; the Gnome::Gtk4::R-CellEditable.
$path; the path identifying the edited cell.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::CellRenderer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.