
Gnome::Gtk4::Grid
Description
Gnome::Gtk4::Grid is a container which arranges its child widgets in rows and columns.
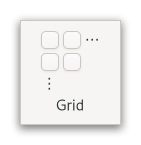
It supports arbitrary positions and horizontal/vertical spans.
Children are added using .attach()
. They can span multiple rows or columns. It is also possible to add a child next to an existing child, using .attach-next-to()
. To remove a child from the grid, use .remove()
.
The behaviour of Gnome::Gtk4::Grid when several children occupy the same grid cell is undefined.
GtkGrid as GtkBuildable
Every child in a Gnome::Gtk4::Grid has access to a custom Gnome::Gtk4::R-Buildable element, called `<layout>`. It can by used to specify a position in the grid and optionally spans. All properties that can be used in the `<layout>` element are implemented by Gnome::Gtk4::GridLayoutChild.
It is implemented by Gnome::Gtk4::Widget using Gnome::Gtk4::LayoutManager.
To showcase it, here is a simple example:
It organizes the first two buttons side-by-side in one cell each. The third button is in the last column but spans across two rows. This is defined by the row-span` property. The last button is located in the second row and spans across two columns, which is defined by the column-span` property.
CSS nodes
Gnome::Gtk4::Grid uses a single CSS node with name grid.
Accessibility
Until GTK 4.10, Gnome::Gtk4::Grid used the GTK_ACCESSIBLE_ROLE_GROUP role.
Starting from GTK 4.12, Gnome::Gtk4::Grid uses the GTK_ACCESSIBLE_ROLE_GENERIC role.
Uml Diagram
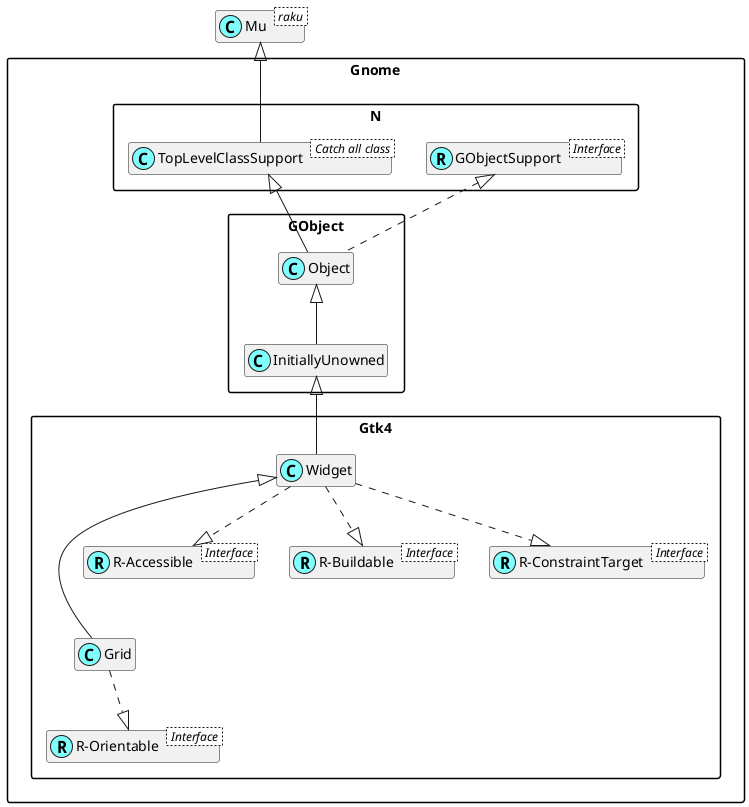
Class initialization
new
:native-object
Create an object using a native object from an object of the same type found elsewhere. See also Gnome::N::TopLevelSupportClass.
multi method new ( N-Object() :$native-object! )
new-grid
Creates a new grid widget.
method new-grid ( --> Gnome::Gtk4::Grid )
Methods
attach
Adds a widget to the grid.
The position of $child
is determined by $column
and $row
. The number of “cells” that $child
will occupy is determined by $width
and $height
.
method attach ( N-Object() $child, Int() $column, Int() $row, Int() $width, Int() $height )
$child; the widget to add.
$column; the column number to attach the left side of
$child
to.$row; the row number to attach the top side of
$child
to.$width; the number of columns that
$child
will span.$height; the number of rows that
$child
will span.
attach-next-to
Adds a widget to the grid.
The widget is placed next to $sibling
, on the side determined by $side
. When $sibling
is undefined, the widget is placed in row (for left or right placement) or column 0 (for top or bottom placement), at the end indicated by $side
.
Attaching widgets labeled `[1]`, `[2]`, `[3]` with ` $sibling
== undefined` and ` $side
== GTK_POS_LEFT
` yields a layout of `[3][2][1]`.
method attach-next-to ( N-Object() $child, N-Object() $sibling, GtkPositionType $side, Int() $width, Int() $height )
$child; the widget to add.
$sibling; the child of
$grid
that$child
will be placed next to, or undefined to place$child
at the beginning or end.$side; the side of
$sibling
that$child
is positioned next to.$width; the number of columns that
$child
will span.$height; the number of rows that
$child
will span.
get-baseline-row
Returns which row defines the global baseline of $grid
.
method get-baseline-row (--> Int )
Return value; the row index defining the global baseline.
get-child-at
Gets the child of $grid
whose area covers the grid cell at $column
, $row
.
method get-child-at ( Int() $column, Int() $row --> N-Object )
$column; the left edge of the cell.
$row; the top edge of the cell.
Return value; the child at the given position.
get-column-homogeneous
Returns whether all columns of $grid
have the same width.
method get-column-homogeneous (--> Bool )
Return value; whether all columns of $grid
have the same width..
get-column-spacing
Returns the amount of space between the columns of $grid
.
method get-column-spacing (--> UInt )
Return value; the column spacing of $grid
.
get-row-baseline-position
Returns the baseline position of $row
.
See .set-row-baseline-position()
.
method get-row-baseline-position ( Int() $row --> GtkBaselinePosition )
$row; a row index.
Return value; the baseline position of $row
.
get-row-homogeneous
Returns whether all rows of $grid
have the same height.
method get-row-homogeneous (--> Bool )
Return value; whether all rows of $grid
have the same height..
get-row-spacing
Returns the amount of space between the rows of $grid
.
method get-row-spacing (--> UInt )
Return value; the row spacing of $grid
.
insert-column
Inserts a column at the specified position.
Children which are attached at or to the right of this position are moved one column to the right. Children which span across this position are grown to span the new column.
method insert-column ( Int() $position )
$position; the position to insert the column at.
insert-next-to
Inserts a row or column at the specified position.
The new row or column is placed next to $sibling
, on the side determined by $side
. If $side
is GTK_POS_TOP
or GTK_POS_BOTTOM
, a row is inserted. If $side
is GTK_POS_LEFT
of GTK_POS_RIGHT
, a column is inserted.
method insert-next-to ( N-Object() $sibling, GtkPositionType $side )
$sibling; the child of
$grid
that the new row or column will be placed next to.$side; the side of
$sibling
that$child
is positioned next to.
insert-row
Inserts a row at the specified position.
Children which are attached at or below this position are moved one row down. Children which span across this position are grown to span the new row.
method insert-row ( Int() $position )
$position; the position to insert the row at.
query-child
Queries the attach points and spans of $child
inside the given Gnome::Gtk4::Grid.
method query-child ( N-Object() $child, Array[Int] $column, Array[Int] $row, Array[Int] $width, Array[Int] $height )
$child; a Gnome::Gtk4::Widget child of
$grid
.$column; (transfer ownership: full) the column used to attach the left side of
$child
.$row; (transfer ownership: full) the row used to attach the top side of
$child
.$width; (transfer ownership: full) the number of columns
$child
spans.$height; (transfer ownership: full) the number of rows
$child
spans.
remove
Removes a child from $grid
.
The child must have been added with .attach()
or .attach-next-to()
.
method remove ( N-Object() $child )
$child; the child widget to remove.
remove-column
Removes a column from the grid.
Children that are placed in this column are removed, spanning children that overlap this column have their width reduced by one, and children after the column are moved to the left.
method remove-column ( Int() $position )
$position; the position of the column to remove.
remove-row
Removes a row from the grid.
Children that are placed in this row are removed, spanning children that overlap this row have their height reduced by one, and children below the row are moved up.
method remove-row ( Int() $position )
$position; the position of the row to remove.
set-baseline-row
Sets which row defines the global baseline for the entire grid.
Each row in the grid can have its own local baseline, but only one of those is global, meaning it will be the baseline in the parent of the $grid
.
method set-baseline-row ( Int() $row )
$row; the row index.
set-column-homogeneous
Sets whether all columns of $grid
will have the same width.
method set-column-homogeneous ( Bool() $homogeneous )
$homogeneous;
True
to make columns homogeneous.
set-column-spacing
Sets the amount of space between columns of $grid
.
method set-column-spacing ( UInt() $spacing )
$spacing; the amount of space to insert between columns.
set-row-baseline-position
Sets how the baseline should be positioned on $row
of the grid, in case that row is assigned more space than is requested.
The default baseline position is GTK_BASELINE_POSITION_CENTER
.
method set-row-baseline-position ( Int() $row, GtkBaselinePosition $pos )
$row; a row index.
$pos; a
enumeration GtkBaselinePosition defined in Gnome::Gtk4::T-enums
.
set-row-homogeneous
Sets whether all rows of $grid
will have the same height.
method set-row-homogeneous ( Bool() $homogeneous )
$homogeneous;
True
to make rows homogeneous.
set-row-spacing
Sets the amount of space between rows of $grid
.
method set-row-spacing ( UInt() $spacing )
$spacing; the amount of space to insert between rows.