
Gnome::Gtk4::TextBuffer
Description
Stores text and attributes for display in a Gnome::Gtk4::TextView.
You may wish to begin by reading the [text widget conceptual overview](section-text-widget.html), which gives an overview of all the objects and data types related to the text widget and how they work together.
GtkTextBuffer can support undoing changes to the buffer content, see .set-enable-undo()
].
Uml Diagram
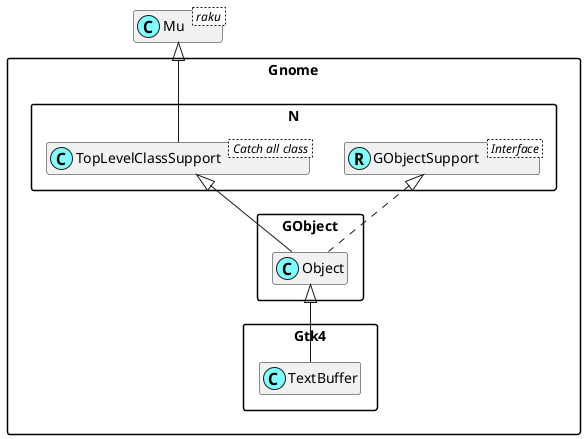
Class initialization
new
:native-object
Create an object using a native object from an object of the same type found elsewhere. See also Gnome::N::TopLevelSupportClass.
multi method new ( N-Object() :$native-object! )
new-textbuffer
Creates a new text buffer.
method new-textbuffer ( N-Object() $table --> Gnome::Gtk4::TextBuffer )
$table; a tag table, or undefined to create a new one.
Methods
add-mark
Adds the mark at position $where
.
The mark must not be added to another buffer, and if its name is defined then there must not be another mark in the buffer with the same name.
Emits the mark-set signal as notification of the mark's initial placement.
method add-mark ( N-Object() $mark, N-Object $where )
$mark; the mark to add.
$where; location to place mark
add-selection-clipboard
Adds $clipboard
to the list of clipboards in which the selection contents of $buffer
are available.
In most cases, $clipboard
will be the Gnome::Gdk4::Clipboard returned by .get-primary-clipboard()
in class Gnome::Gtk4::Widget] for a view of $buffer
.
method add-selection-clipboard ( N-Object() $clipboard )
$clipboard; a Gnome::Gdk4::Clipboard.
apply-tag
Emits the “apply-tag” signal on $buffer
.
The default handler for the signal applies $tag
to the given range. $start
and $end
do not have to be in order.
method apply-tag ( N-Object() $tag, N-Object $start, N-Object $end )
$tag; a Gnome::Gtk4::TextTag.
$start; one bound of range to be tagged
$end; other bound of range to be tagged
apply-tag-by-name
Emits the “apply-tag” signal on $buffer
.
Calls .lookup()
in class Gnome::Gtk4::TextTagTable] on the buffer’s tag table to get a Gnome::Gtk4::TextTag, then calls .apply-tag()
].
method apply-tag-by-name ( Str $name, N-Object $start, N-Object $end )
$name; name of a named Gnome::Gtk4::TextTag.
$start; one bound of range to be tagged
$end; other bound of range to be tagged
backspace
Performs the appropriate action as if the user hit the delete key with the cursor at the position specified by $iter
.
In the normal case a single character will be deleted, but when combining accents are involved, more than one character can be deleted, and when precomposed character and accent combinations are involved, less than one character will be deleted.
Because the buffer is modified, all outstanding iterators become invalid after calling this function; however, the $iter
will be re-initialized to point to the location where text was deleted.
method backspace ( N-Object $iter, Bool() $interactive, Bool() $default-editable --> Bool )
$iter; a position in
$buffer
$interactive; whether the deletion is caused by user interaction.
$default-editable; whether the buffer is editable by default.
Return value; True
if the buffer was modified.
begin-irreversible-action
Denotes the beginning of an action that may not be undone.
This will cause any previous operations in the undo/redo queue to be cleared.
This should be paired with a call to .end-irreversible-action()
] after the irreversible action has completed.
You may nest calls to .begin-irreversible-action()
and .end-irreversible-action()
pairs.
method begin-irreversible-action ( )
begin-user-action
Called to indicate that the buffer operations between here and a call to .end-user-action()
are part of a single user-visible operation.
The operations between .begin-user-action()
and .end-user-action()
can then be grouped when creating an undo stack. Gnome::Gtk4::TextBuffer maintains a count of calls to .begin-user-action()
that have not been closed with a call to .end-user-action()
, and emits the “begin-user-action” and “end-user-action” signals only for the outermost pair of calls. This allows you to build user actions from other user actions.
The “interactive” buffer mutation functions, such as .insert-interactive()
], automatically call begin/end user action around the buffer operations they perform, so there's no need to add extra calls if you user action consists solely of a single call to one of those functions.
method begin-user-action ( )
copy-clipboard
Copies the currently-selected text to a clipboard.
method copy-clipboard ( N-Object() $clipboard )
$clipboard; the Gnome::Gdk4::Clipboard object to copy to.
create-child-anchor
Creates and inserts a child anchor.
This is a convenience function which simply creates a child anchor with .newtextbuffer()
in class Gnome::Gtk4::TextChildAnchor and inserts it into the buffer with .insert-child-anchor()
].
The new anchor is owned by the buffer; no reference count is returned to the caller of this function.
method create-child-anchor ( N-Object $iter --> N-Object )
$iter; location in the buffer
Return value; the created child anchor.
create-mark
Creates a mark at position $where
.
If $mark-name
is undefined, the mark is anonymous; otherwise, the mark can be retrieved by name using .get-mark()
]. If a mark has left gravity, and text is inserted at the mark’s current location, the mark will be moved to the left of the newly-inserted text. If the mark has right gravity ( $left-gravity
= False
), the mark will end up on the right of newly-inserted text. The standard left-to-right cursor is a mark with right gravity (when you type, the cursor stays on the right side of the text you’re typing).
The caller of this function does not own a reference to the returned Gnome::Gtk4::TextMark, so you can ignore the return value if you like. Marks are owned by the buffer and go away when the buffer does.
Emits the mark-set signal as notification of the mark's initial placement.
method create-mark ( Str $mark-name, N-Object $where, Bool() $left-gravity --> N-Object )
$mark-name; name for mark.
$where; location to place mark
$left-gravity; whether the mark has left gravity.
Return value; the new Gnome::Gtk4::TextMark object.
create-tag This function is not yet available
Creates a tag and adds it to the tag table for $buffer
.
Equivalent to calling .newtextbuffer()
in class Gnome::Gtk4::TextTag and then adding the tag to the buffer’s tag table. The returned tag is owned by the buffer’s tag table, so the ref count will be equal to one.
If $tag-name
is undefined, the tag is anonymous.
If $tag-name
is non-undefined, a tag called $tag-name
must not already exist in the tag table for this buffer.
The $first-property-name
argument and subsequent arguments are a list of properties to set on the tag, as with g_object_set().
method create-tag ( Str $tag-name, Str $first-property-name, … --> N-Object )
$tag-name; name of the new tag.
$first-property-name; name of first property to set.
…; …. Note that each argument must be specified as a type followed by its value!
Return value; a new tag.
cut-clipboard
Copies the currently-selected text to a clipboard, then deletes said text if it’s editable.
method cut-clipboard ( N-Object() $clipboard, Bool() $default-editable )
$clipboard; the Gnome::Gdk4::Clipboard object to cut to.
$default-editable; default editability of the buffer.
delete
Deletes text between $start
and $end
.
The order of $start
and $end
is not actually relevant; .delete()
will reorder them.
This function actually emits the “delete-range” signal, and the default handler of that signal deletes the text. Because the buffer is modified, all outstanding iterators become invalid after calling this function; however, the $start
and $end
will be re-initialized to point to the location where text was deleted.
method delete ( N-Object $start, N-Object $end )
$start; a position in
$buffer
$end; another position in
$buffer
delete-interactive
Deletes all editable text in the given range.
Calls .delete()
] for each editable sub-range of [ $start
, $end
). $start
and $end
are revalidated to point to the location of the last deleted range, or left untouched if no text was deleted.
method delete-interactive ( N-Object $start-iter, N-Object $end-iter, Bool() $default-editable --> Bool )
$start-iter; start of range to delete
$end-iter; end of range
$default-editable; whether the buffer is editable by default.
Return value; whether some text was actually deleted.
delete-mark
Deletes $mark
, so that it’s no longer located anywhere in the buffer.
Removes the reference the buffer holds to the mark, so if you haven’t called g_object_ref() on the mark, it will be freed. Even if the mark isn’t freed, most operations on $mark
become invalid, until it gets added to a buffer again with .add-mark()
]. Use .get-deleted()
in class Gnome::Gtk4::TextMark] to find out if a mark has been removed from its buffer.
The mark-deleted signal will be emitted as notification after the mark is deleted.
method delete-mark ( N-Object() $mark )
$mark; a Gnome::Gtk4::TextMark in
$buffer
.
delete-mark-by-name
Deletes the mark named $name
; the mark must exist.
See .delete-mark()
] for details.
method delete-mark-by-name ( Str $name )
$name; name of a mark in
$buffer
.
delete-selection
Deletes the range between the “insert” and “selection_bound” marks, that is, the currently-selected text.
If $interactive
is True
, the editability of the selection will be considered (users can’t delete uneditable text).
method delete-selection ( Bool() $interactive, Bool() $default-editable --> Bool )
$interactive; whether the deletion is caused by user interaction.
$default-editable; whether the buffer is editable by default.
Return value; whether there was a non-empty selection to delete.
end-irreversible-action
Denotes the end of an action that may not be undone.
This will cause any previous operations in the undo/redo queue to be cleared.
This should be called after completing modifications to the text buffer after .begin-irreversible-action()
] was called.
You may nest calls to .begin-irreversible-action()
and .end-irreversible-action()
pairs.
method end-irreversible-action ( )
end-user-action
Ends a user-visible operation.
Should be paired with a call to .begin-user-action()
]. See that function for a full explanation.
method end-user-action ( )
get-bounds
Retrieves the first and last iterators in the buffer, i.e. the entire buffer lies within the range [ $start
, $end
).
method get-bounds ( N-Object $start, N-Object $end )
$start; iterator to initialize with first position in the buffer
$end; iterator to initialize with the end iterator
get-can-redo
Gets whether there is a redoable action in the history.
method get-can-redo (--> Bool )
Return value; True
if there is a redoable action.
get-can-undo
Gets whether there is an undoable action in the history.
method get-can-undo (--> Bool )
Return value; True
if there is an undoable action.
get-char-count
Gets the number of characters in the buffer.
Note that characters and bytes are not the same, you can’t e.g. expect the contents of the buffer in string form to be this many bytes long.
The character count is cached, so this function is very fast.
method get-char-count (--> Int )
Return value; number of characters in the buffer.
get-enable-undo
Gets whether the buffer is saving modifications to the buffer to allow for undo and redo actions.
See .begin-irreversible-action()
] and .end-irreversible-action()
] to create changes to the buffer that cannot be undone.
method get-enable-undo (--> Bool )
Return value; True
if undoing and redoing changes to the buffer is allowed..
get-end-iter
Initializes $iter
with the “end iterator,” one past the last valid character in the text buffer.
If dereferenced with .get-char()
in class Gnome::Gtk4::N-TextIter], the end iterator has a character value of 0. The entire buffer lies in the range from the first position in the buffer (call .get-start-iter()
] to get character position 0) to the end iterator.
method get-end-iter ( N-Object $iter )
$iter; iterator to initialize
get-has-selection
Indicates whether the buffer has some text currently selected.
method get-has-selection (--> Bool )
Return value; True
if the there is text selected.
get-insert
Returns the mark that represents the cursor (insertion point).
Equivalent to calling .get-mark()
] to get the mark named “insert”, but very slightly more efficient, and involves less typing.
method get-insert (--> N-Object )
Return value; insertion point mark.
get-iter-at-child-anchor
Obtains the location of $anchor
within $buffer
.
method get-iter-at-child-anchor ( N-Object $iter, N-Object() $anchor )
$iter; an iterator to be initialized
$anchor; a child anchor that appears in
$buffer
.
get-iter-at-line
Initializes $iter
to the start of the given line.
If $line-number
is greater than or equal to the number of lines in the $buffer
, the end iterator is returned.
method get-iter-at-line ( N-Object $iter, Int() $line-number --> Bool )
$iter; iterator to initialize
$line-number; line number counting from 0.
Return value; whether the exact position has been found.
get-iter-at-line-index
Obtains an iterator pointing to $byte-index
within the given line. $byte-index
must be the start of a UTF-8 character. Note bytes, not characters; UTF-8 may encode one character as multiple bytes.
If $line-number
is greater than or equal to the number of lines in the $buffer
, the end iterator is returned. And if $byte-index
is off the end of the line, the iterator at the end of the line is returned.
method get-iter-at-line-index ( N-Object $iter, Int() $line-number, Int() $byte-index --> Bool )
$iter; iterator to initialize
$line-number; line number counting from 0.
$byte-index; byte index from start of line.
Return value; whether the exact position has been found.
get-iter-at-line-offset
Obtains an iterator pointing to $char-offset
within the given line.
Note characters, not bytes; UTF-8 may encode one character as multiple bytes.
If $line-number
is greater than or equal to the number of lines in the $buffer
, the end iterator is returned. And if $char-offset
is off the end of the line, the iterator at the end of the line is returned.
method get-iter-at-line-offset ( N-Object $iter, Int() $line-number, Int() $char-offset --> Bool )
$iter; iterator to initialize
$line-number; line number counting from 0.
$char-offset; char offset from start of line.
Return value; whether the exact position has been found.
get-iter-at-mark
Initializes $iter
with the current position of $mark
.
method get-iter-at-mark ( N-Object $iter, N-Object() $mark )
$iter; iterator to initialize
$mark; a Gnome::Gtk4::TextMark in
$buffer
.
get-iter-at-offset
Initializes $iter
to a position $char-offset
chars from the start of the entire buffer.
If $char-offset
is -1 or greater than the number of characters in the buffer, $iter
is initialized to the end iterator, the iterator one past the last valid character in the buffer.
method get-iter-at-offset ( N-Object $iter, Int() $char-offset )
$iter; iterator to initialize
$char-offset; char offset from start of buffer, counting from 0, or -1.
get-line-count
Obtains the number of lines in the buffer.
This value is cached, so the function is very fast.
method get-line-count (--> Int )
Return value; number of lines in the buffer.
get-mark
Returns the mark named $name
in buffer $buffer
, or undefined if no such mark exists in the buffer.
method get-mark ( Str $name --> N-Object )
$name; a mark name.
Return value; a Gnome::Gtk4::TextMark.
get-max-undo-levels
Gets the maximum number of undo levels to perform.
If 0, unlimited undo actions may be performed. Note that this may have a memory usage impact as it requires storing an additional copy of the inserted or removed text within the text buffer.
method get-max-undo-levels (--> UInt )
Return value; The max number of undo levels allowed (0 indicates unlimited)..
get-modified
Indicates whether the buffer has been modified since the last call to .set-modified()
] set the modification flag to False
.
Used for example to enable a “save” function in a text editor.
method get-modified (--> Bool )
Return value; True
if the buffer has been modified.
get-selection-bound
Returns the mark that represents the selection bound.
Equivalent to calling .get-mark()
] to get the mark named “selection_bound”, but very slightly more efficient, and involves less typing.
The currently-selected text in $buffer
is the region between the “selection_bound” and “insert” marks. If “selection_bound” and “insert” are in the same place, then there is no current selection. .get-selection-bounds()
] is another convenient function for handling the selection, if you just want to know whether there’s a selection and what its bounds are.
method get-selection-bound (--> N-Object )
Return value; selection bound mark.
get-selection-bounds
Returns True
if some text is selected; places the bounds of the selection in $start
and $end
.
If the selection has length 0, then $start
and $end
are filled in with the same value. $start
and $end
will be in ascending order. If $start
and $end
are undefined, then they are not filled in, but the return value still indicates whether text is selected.
method get-selection-bounds ( N-Object $start, N-Object $end --> Bool )
$start; iterator to initialize with selection start
$end; iterator to initialize with selection end
Return value; whether the selection has nonzero length.
get-selection-content
Get a content provider for this buffer.
It can be used to make the content of $buffer
available in a Gnome::Gdk4::Clipboard, see .set-content()
in class Gnome::Gdk4::Clipboard].
method get-selection-content (--> N-Object )
Return value; a new Gnome::Gdk4::ContentProvider..
get-slice
Returns the text in the range [ $start
, $end
).
Excludes undisplayed text (text marked with tags that set the invisibility attribute) if $include-hidden-chars
is False
. The returned string includes a 0xFFFC character whenever the buffer contains embedded images, so byte and character indexes into the returned string do correspond to byte and character indexes into the buffer. Contrast with .get-text()
]. Note that 0xFFFC can occur in normal text as well, so it is not a reliable indicator that a paintable or widget is in the buffer.
method get-slice ( N-Object $start, N-Object $end, Bool() $include-hidden-chars --> Str )
$start; start of a range
$end; end of a range
$include-hidden-chars; whether to include invisible text.
Return value; an allocated UTF-8 string.
get-start-iter
Initialized $iter
with the first position in the text buffer.
This is the same as using .get-iter-at-offset()
] to get the iter at character offset 0.
method get-start-iter ( N-Object $iter )
$iter; iterator to initialize
get-tag-table
Get the Gnome::Gtk4::TextTagTable associated with this buffer.
method get-tag-table (--> N-Object )
Return value; the buffer’s tag table.
get-text
Returns the text in the range [ $start
, $end
).
Excludes undisplayed text (text marked with tags that set the invisibility attribute) if $include-hidden-chars
is False
. Does not include characters representing embedded images, so byte and character indexes into the returned string do not correspond to byte and character indexes into the buffer. Contrast with .get-slice()
].
method get-text ( N-Object $start, N-Object $end, Bool() $include-hidden-chars --> Str )
$start; start of a range
$end; end of a range
$include-hidden-chars; whether to include invisible text.
Return value; an allocated UTF-8 string.
insert
Inserts $len
bytes of $text
at position $iter
.
If $len
is -1, $text
must be nul-terminated and will be inserted in its entirety. Emits the “insert-text” signal; insertion actually occurs in the default handler for the signal. $iter
is invalidated when insertion occurs (because the buffer contents change), but the default signal handler revalidates it to point to the end of the inserted text.
method insert ( N-Object $iter, Str $text, Int() $len )
$iter; a position in the buffer
$text; text in UTF-8 format.
$len; length of text in bytes, or -1.
insert-at-cursor
Inserts $text
in $buffer
.
Simply calls .insert()
], using the current cursor position as the insertion point.
method insert-at-cursor ( Str $text, Int() $len )
$text; text in UTF-8 format.
$len; length of text, in bytes.
insert-child-anchor
Inserts a child widget anchor into the text buffer at $iter
.
The anchor will be counted as one character in character counts, and when obtaining the buffer contents as a string, will be represented by the Unicode “object replacement character” 0xFFFC. Note that the “slice” variants for obtaining portions of the buffer as a string include this character for child anchors, but the “text” variants do not. E.g. see .get-slice()
] and .get-text()
].
Consider .create-child-anchor()
] as a more convenient alternative to this function. The buffer will add a reference to the anchor, so you can unref it after insertion.
method insert-child-anchor ( N-Object $iter, N-Object() $anchor )
$iter; location to insert the anchor
$anchor; a Gnome::Gtk4::TextChildAnchor.
insert-interactive
Inserts $text
in $buffer
.
Like .insert()
], but the insertion will not occur if $iter
is at a non-editable location in the buffer. Usually you want to prevent insertions at ineditable locations if the insertion results from a user action (is interactive). $default-editable
indicates the editability of text that doesn't have a tag affecting editability applied to it. Typically the result of .get-editable()
in class Gnome::Gtk4::TextView] is appropriate here.
method insert-interactive ( N-Object $iter, Str $text, Int() $len, Bool() $default-editable --> Bool )
$iter; a position in
$buffer
$text; some UTF-8 text.
$len; length of text in bytes, or -1.
$default-editable; default editability of buffer.
Return value; whether text was actually inserted.
insert-interactive-at-cursor
Inserts $text
in $buffer
.
Calls .insert-interactive()
] at the cursor position. $default-editable
indicates the editability of text that doesn't have a tag affecting editability applied to it. Typically the result of .get-editable()
in class Gnome::Gtk4::TextView] is appropriate here.
method insert-interactive-at-cursor ( Str $text, Int() $len, Bool() $default-editable --> Bool )
$text; text in UTF-8 format.
$len; length of text in bytes, or -1.
$default-editable; default editability of buffer.
Return value; whether text was actually inserted.
insert-markup
Inserts the text in $markup
at position $iter
. $markup
will be inserted in its entirety and must be nul-terminated and valid UTF-8. Emits the insert-text signal, possibly multiple times; insertion actually occurs in the default handler for the signal. $iter
will point to the end of the inserted text on return.
method insert-markup ( N-Object $iter, Str $markup, Int() $len )
$iter; location to insert the markup
$markup; a nul-terminated UTF-8 string containing Pango markup.
$len; length of
$markup
in bytes, or -1.
insert-paintable
Inserts an image into the text buffer at $iter
.
The image will be counted as one character in character counts, and when obtaining the buffer contents as a string, will be represented by the Unicode “object replacement character” 0xFFFC. Note that the “slice” variants for obtaining portions of the buffer as a string include this character for paintable, but the “text” variants do not. e.g. see .get-slice()
] and .get-text()
].
method insert-paintable ( N-Object $iter, N-Object() $paintable )
$iter; location to insert the paintable
$paintable; a Gnome::Gdk4::R-Paintable.
insert-range
Copies text, tags, and paintables between $start
and $end
and inserts the copy at $iter
.
The order of $start
and $end
doesn’t matter.
Used instead of simply getting/inserting text because it preserves images and tags. If $start
and $end
are in a different buffer from $buffer
, the two buffers must share the same tag table.
Implemented via emissions of the insert-text and apply-tag signals, so expect those.
method insert-range ( N-Object $iter, N-Object $start, N-Object $end )
$iter; a position in
$buffer
$start; a position in a Gnome::Gtk4::TextBuffer
$end; another position in the same buffer as
$start
insert-range-interactive
Copies text, tags, and paintables between $start
and $end
and inserts the copy at $iter
.
Same as .insert-range()
], but does nothing if the insertion point isn’t editable. The $default-editable
parameter indicates whether the text is editable at $iter
if no tags enclosing $iter
affect editability. Typically the result of .get-editable()
in class Gnome::Gtk4::TextView] is appropriate here.
method insert-range-interactive ( N-Object $iter, N-Object $start, N-Object $end, Bool() $default-editable --> Bool )
$iter; a position in
$buffer
$start; a position in a Gnome::Gtk4::TextBuffer
$end; another position in the same buffer as
$start
$default-editable; default editability of the buffer.
Return value; whether an insertion was possible at $iter
.
insert-with-tags This function is not yet available
Inserts $text
into $buffer
at $iter
, applying the list of tags to the newly-inserted text.
The last tag specified must be undefined to terminate the list. Equivalent to calling .insert()
], then .apply-tag()
] on the inserted text; this is just a convenience function.
method insert-with-tags ( N-Object $iter, Str $text, Int() $len, N-Object() $first-tag, … )
$iter; an iterator in
$buffer
$text; UTF-8 text.
$len; length of
$text
, or -1.$first-tag; first tag to apply to
$text
.…; …. Note that each argument must be specified as a type followed by its value!
insert-with-tags-by-name This function is not yet available
Inserts $text
into $buffer
at $iter
, applying the list of tags to the newly-inserted text.
Same as .insert-with-tags()
], but allows you to pass in tag names instead of tag objects.
method insert-with-tags-by-name ( N-Object $iter, Str $text, Int() $len, Str $first-tag-name, … )
$iter; position in
$buffer
$text; UTF-8 text.
$len; length of
$text
, or -1.$first-tag-name; name of a tag to apply to
$text
.…; …. Note that each argument must be specified as a type followed by its value!
move-mark
Moves $mark
to the new location $where
.
Emits the mark-set signal as notification of the move.
method move-mark ( N-Object() $mark, N-Object $where )
$mark; a Gnome::Gtk4::TextMark.
$where; new location for
$mark
in$buffer
move-mark-by-name
Moves the mark named $name
(which must exist) to location $where
.
See .move-mark()
] for details.
method move-mark-by-name ( Str $name, N-Object $where )
$name; name of a mark.
$where; new location for mark
paste-clipboard
Pastes the contents of a clipboard.
If $override-location
is undefined, the pasted text will be inserted at the cursor position, or the buffer selection will be replaced if the selection is non-empty.
Note: pasting is asynchronous, that is, we’ll ask for the paste data and return, and at some point later after the main loop runs, the paste data will be inserted.
method paste-clipboard ( N-Object() $clipboard, N-Object $override-location, Bool() $default-editable )
$clipboard; the Gnome::Gdk4::Clipboard to paste from.
$override-location; location to insert pasted text
$default-editable; whether the buffer is editable by default.
place-cursor
This function moves the “insert” and “selection_bound” marks simultaneously.
If you move them to the same place in two steps with .move-mark()
], you will temporarily select a region in between their old and new locations, which can be pretty inefficient since the temporarily-selected region will force stuff to be recalculated. This function moves them as a unit, which can be optimized.
method place-cursor ( N-Object $where )
$where; where to put the cursor
redo
Redoes the next redoable action on the buffer, if there is one.
method redo ( )
remove-all-tags
Removes all tags in the range between $start
and $end
.
Be careful with this function; it could remove tags added in code unrelated to the code you’re currently writing. That is, using this function is probably a bad idea if you have two or more unrelated code sections that add tags.
method remove-all-tags ( N-Object $start, N-Object $end )
$start; one bound of range to be untagged
$end; other bound of range to be untagged
remove-selection-clipboard
Removes a Gnome::Gdk4::Clipboard added with .add-selection-clipboard()
]
method remove-selection-clipboard ( N-Object() $clipboard )
$clipboard; a Gnome::Gdk4::Clipboard added to
$buffer
by.add-selection-clipboard()
].
remove-tag
Emits the “remove-tag” signal.
The default handler for the signal removes all occurrences of $tag
from the given range. $start
and $end
don’t have to be in order.
method remove-tag ( N-Object() $tag, N-Object $start, N-Object $end )
$tag; a Gnome::Gtk4::TextTag.
$start; one bound of range to be untagged
$end; other bound of range to be untagged
remove-tag-by-name
Emits the “remove-tag” signal.
Calls .lookup()
in class Gnome::Gtk4::TextTagTable] on the buffer’s tag table to get a Gnome::Gtk4::TextTag, then calls .remove-tag()
].
method remove-tag-by-name ( Str $name, N-Object $start, N-Object $end )
$name; name of a Gnome::Gtk4::TextTag.
$start; one bound of range to be untagged
$end; other bound of range to be untagged
select-range
This function moves the “insert” and “selection_bound” marks simultaneously.
If you move them in two steps with .move-mark()
], you will temporarily select a region in between their old and new locations, which can be pretty inefficient since the temporarily-selected region will force stuff to be recalculated. This function moves them as a unit, which can be optimized.
method select-range ( N-Object $ins, N-Object $bound )
$ins; where to put the “insert” mark
$bound; where to put the “selection_bound” mark
set-enable-undo
Sets whether or not to enable undoable actions in the text buffer.
Undoable actions in this context are changes to the text content of the buffer. Changes to tags and marks are not tracked.
If enabled, the user will be able to undo the last number of actions up to .get-max-undo-levels()
].
See .begin-irreversible-action()
] and .end-irreversible-action()
] to create changes to the buffer that cannot be undone.
method set-enable-undo ( Bool() $enable-undo )
$enable-undo;
True
to enable undo.
set-max-undo-levels
Sets the maximum number of undo levels to perform.
If 0, unlimited undo actions may be performed. Note that this may have a memory usage impact as it requires storing an additional copy of the inserted or removed text within the text buffer.
method set-max-undo-levels ( UInt() $max-undo-levels )
$max-undo-levels; the maximum number of undo actions to perform.
set-modified
Used to keep track of whether the buffer has been modified since the last time it was saved.
Whenever the buffer is saved to disk, call gtk_text_buffer_set_modified ( $buffer
, FALSE)`. When the buffer is modified, it will automatically toggle on the modified bit again. When the modified bit flips, the buffer emits the modified-changed signal.
method set-modified ( Bool() $setting )
$setting; modification flag setting.
set-text
Deletes current contents of $buffer
, and inserts $text
instead. This is automatically marked as an irreversible action in the undo stack. If you wish to mark this action as part of a larger undo operation, call [method $TextBuffer
.delete] and [method $TextBuffer
.insert] directly instead.
If $len
is -1, $text
must be nul-terminated. $text
must be valid UTF-8.
method set-text ( Str $text, Int() $len )
$text; UTF-8 text to insert.
$len; length of
$text
in bytes.
undo
Undoes the last undoable action on the buffer, if there is one.
method undo ( )
Signals
apply-tag
Emitted to apply a tag to a range of text in a Gnome::Gtk4::TextBuffer.
Applying actually occurs in the default handler.
Note that if your handler runs before the default handler it must not invalidate the $start
and $end
iters (or has to revalidate them).
See also: .apply-tag()
], .insert-with-tags()
], .insert-range()
].
method handler ( N-Object $tag, N-Object $start, N-Object $end, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$tag; the applied tag.
$start; the start of the range the tag is applied to.
$end; the end of the range the tag is applied to.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
begin-user-action
Emitted at the beginning of a single user-visible operation on a Gnome::Gtk4::TextBuffer.
See also: .begin-user-action()
], .insert-interactive()
], .insert-range-interactive()
], .delete-interactive()
], .backspace()
], .delete-selection()
].
method handler ( Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
changed
Emitted when the content of a Gnome::Gtk4::TextBuffer has changed.
method handler ( Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
delete-range
Emitted to delete a range from a Gnome::Gtk4::TextBuffer.
Note that if your handler runs before the default handler it must not invalidate the $start
and $end
iters (or has to revalidate them). The default signal handler revalidates the $start
and $end
iters to both point to the location where text was deleted. Handlers which run after the default handler (see g_signal_connect_after()) do not have access to the deleted text.
See also: .delete()
].
method handler ( N-Object $start, N-Object $end, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$start; the start of the range to be deleted.
$end; the end of the range to be deleted.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
end-user-action
Emitted at the end of a single user-visible operation on the Gnome::Gtk4::TextBuffer.
See also: .end-user-action()
], .insert-interactive()
], .insert-range-interactive()
], .delete-interactive()
], .backspace()
], .delete-selection()
], .backspace()
].
method handler ( Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
insert-child-anchor
Emitted to insert a Gnome::Gtk4::TextChildAnchor in a Gnome::Gtk4::TextBuffer.
Insertion actually occurs in the default handler.
Note that if your handler runs before the default handler it must not invalidate the $location
iter (or has to revalidate it). The default signal handler revalidates it to be placed after the inserted $anchor
.
See also: .insert-child-anchor()
].
method handler ( N-Object $location, N-Object $anchor, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$location; position to insert
$anchor
in$textbuffer
.$anchor; the Gnome::Gtk4::TextChildAnchor to be inserted.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
insert-paintable
Emitted to insert a Gnome::Gdk4::R-Paintable in a Gnome::Gtk4::TextBuffer.
Insertion actually occurs in the default handler.
Note that if your handler runs before the default handler it must not invalidate the $location
iter (or has to revalidate it). The default signal handler revalidates it to be placed after the inserted $paintable
.
See also: .insert-paintable()
].
method handler ( N-Object $location, $paintable, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$location; position to insert
$paintable
in$textbuffer
.$paintable; the Gnome::Gdk4::R-Paintable to be inserted.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
insert-text
Emitted to insert text in a Gnome::Gtk4::TextBuffer.
Insertion actually occurs in the default handler.
Note that if your handler runs before the default handler it must not invalidate the $location
iter (or has to revalidate it). The default signal handler revalidates it to point to the end of the inserted text.
See also: .insert()
], .insert-range()
].
method handler ( N-Object $location, Str $text, gint $len, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$location; position to insert
$text
in$textbuffer
.$text; the UTF-8 text to be inserted.
$len; length of the inserted text in bytes.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
mark-deleted
Emitted as notification after a Gnome::Gtk4::TextMark is deleted.
See also: .delete-mark()
].
method handler ( N-Object $mark, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$mark; The mark that was deleted.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
mark-set
Emitted as notification after a Gnome::Gtk4::TextMark is set.
See also: .create-mark()
], .move-mark()
].
method handler ( N-Object $location, N-Object $mark, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$location; The location of
$mark
in$textbuffer
.$mark; The mark that is set.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
modified-changed
Emitted when the modified bit of a Gnome::Gtk4::TextBuffer flips.
See also: .set-modified()
].
method handler ( Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
paste-done
Emitted after paste operation has been completed.
This is useful to properly scroll the view to the end of the pasted text. See .paste-clipboard()
] for more details.
method handler ( $clipboard, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$clipboard; the Gnome::Gdk4::Clipboard pasted from.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
redo
Emitted when a request has been made to redo the previously undone operation.
method handler ( Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
remove-tag
Emitted to remove all occurrences of $tag
from a range of text in a Gnome::Gtk4::TextBuffer.
Removal actually occurs in the default handler.
Note that if your handler runs before the default handler it must not invalidate the $start
and $end
iters (or has to revalidate them).
See also: .remove-tag()
].
method handler ( N-Object $tag, N-Object $start, N-Object $end, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$tag; the tag to be removed.
$start; the start of the range the tag is removed from.
$end; the end of the range the tag is removed from.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
undo
Emitted when a request has been made to undo the previous operation or set of operations that have been grouped together.
method handler ( Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::TextBuffer :$_widget, *C<user>-options )
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::TextBuffer object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.