
Gnome::Gtk4::Image
Description
The Gnome::Gtk4::Image widget displays an image.
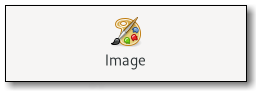
Various kinds of object can be displayed as an image; most typically, you would load a Gnome::Gdk4::Texture from a file, using the convenience function .new-from-file()
, for instance:
If the file isn’t loaded successfully, the image will contain a “broken image” icon similar to that used in many web browsers.
If you want to handle errors in loading the file yourself, for example by displaying an error message, then load the image with .new-from-file() in class Gnome::Gdk4::Texture
, then create the Gnome::Gtk4::Image with .new-from-paintable()
.
Sometimes an application will want to avoid depending on external data files, such as image files. See the documentation of Gnome::Gio::N-Resource inside GIO, for details. In this case, resource, .new-from-resource()
, and .set-from-resource()
should be used.
Gnome::Gtk4::Image displays its image as an icon, with a size that is determined by the application. See Gnome::Gtk4::Picture if you want to show an image at is actual size.
CSS nodes
Gnome::Gtk4::Image has a single CSS node with the name image. The style classes `.normal-icons` or `.large-icons` may appear, depending on the icon-size property.
Accessibility
Gnome::Gtk4::Image uses the GTK_ACCESSIBLE_ROLE_IMG role.
Class initialization
new
:native-object
Create an object using a native object from elsewhere. See also Gnome::N::TopLevelSupportClass.
multi method new ( N-Object :$native-object! )
new-image
Creates a new empty Gnome::Gtk4::Image widget.
method new-image ( --> Gnome::Gtk4::Image \)
new-from-file
Creates a new Gnome::Gtk4::Image displaying the file $filename
.
If the file isn’t found or can’t be loaded, the resulting Gnome::Gtk4::Image will display a “broken image” icon. This function never returns undefined, it always returns a valid Gnome::Gtk4::Image widget.
If you need to detect failures to load the file, use .new-from-file() in class Gnome::Gdk4::Texture
to load the file yourself, then create the Gnome::Gtk4::Image from the texture.
The storage type (see .get-storage-type()
) of the returned image is not defined, it will be whatever is appropriate for displaying the file.
method new-from-file ( Str $filename --> Gnome::Gtk4::Image \)
$filename; a filename.
new-from-gicon
Creates a Gnome::Gtk4::Image displaying an icon from the current icon theme.
If the icon name isn’t known, a “broken image” icon will be displayed instead. If the current icon theme is changed, the icon will be updated appropriately.
method new-from-gicon ( N-Object() $icon --> Gnome::Gtk4::Image \)
$icon; an icon.
new-from-icon-name
Creates a Gnome::Gtk4::Image displaying an icon from the current icon theme.
If the icon name isn’t known, a “broken image” icon will be displayed instead. If the current icon theme is changed, the icon will be updated appropriately.
method new-from-icon-name ( Str $icon-name --> Gnome::Gtk4::Image \)
$icon-name; an icon name.
new-from-paintable
Creates a new Gnome::Gtk4::Image displaying $paintable
.
The Gnome::Gtk4::Image does not assume a reference to the paintable; you still need to unref it if you own references. Gnome::Gtk4::Image will add its own reference rather than adopting yours.
The Gnome::Gtk4::Image will track changes to the $paintable
and update its size and contents in response to it.
method new-from-paintable ( N-Object() $paintable --> Gnome::Gtk4::Image \)
$paintable; a Gnome::Gdk4::R-Paintable.
new-from-pixbuf
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.12
Creates a new Gnome::Gtk4::Image displaying $pixbuf
.
The Gnome::Gtk4::Image does not assume a reference to the pixbuf; you still need to unref it if you own references. Gnome::Gtk4::Image will add its own reference rather than adopting yours.
This is a helper for .new-from-paintable()
, and you can't get back the exact pixbuf once this is called, only a texture.
Note that this function just creates an Gnome::Gtk4::Image from the pixbuf. The Gnome::Gtk4::Image created will not react to state changes. Should you want that, you should use .new-from-icon-name()
.
method new-from-pixbuf ( N-Object() $pixbuf --> Gnome::Gtk4::Image \)
$pixbuf; a Gnome::GdkPixbuf::Pixbuf.
new-from-resource
Creates a new Gnome::Gtk4::Image displaying the resource file $resource-path
.
If the file isn’t found or can’t be loaded, the resulting Gnome::Gtk4::Image will display a “broken image” icon. This function never returns undefined, it always returns a valid Gnome::Gtk4::Image widget.
If you need to detect failures to load the file, use .new-from-file() in class Gnome::Gtk4::Image
to load the file yourself, then create the Gnome::Gtk4::Image from the pixbuf.
The storage type (see .get-storage-type()
) of the returned image is not defined, it will be whatever is appropriate for displaying the file.
method new-from-resource ( Str $resource-path --> Gnome::Gtk4::Image \)
$resource-path; a resource path.
Methods
clear
Resets the image to be empty.
method clear ( )
get-gicon
Gets the Gnome::Gio::R-Icon being displayed by the Gnome::Gtk4::Image.
The storage type of the image must be GTK_IMAGE_EMPTY
or GTK_IMAGE_GICON
(see .get-storage-type()
). The caller of this function does not own a reference to the returned Gnome::Gio::R-Icon.
method get-gicon (--> N-Object )
Return value; a Gnome::Gio::R-Icon.
get-icon-name
Gets the icon name and size being displayed by the Gnome::Gtk4::Image.
The storage type of the image must be GTK_IMAGE_EMPTY
or GTK_IMAGE_ICON_NAME
(see .get-storage-type()
). The returned string is owned by the Gnome::Gtk4::Image and should not be freed.
method get-icon-name (--> Str )
Return value; the icon name.
get-icon-size
Gets the icon size used by the $image
when rendering icons.
method get-icon-size (--> GtkIconSize )
Return value; the image size used by icons.
get-paintable
Gets the image Gnome::Gdk4::R-Paintable being displayed by the Gnome::Gtk4::Image.
The storage type of the image must be GTK_IMAGE_EMPTY
or GTK_IMAGE_PAINTABLE
(see .get-storage-type()
). The caller of this function does not own a reference to the returned paintable.
method get-paintable (--> N-Object )
Return value; the displayed paintable.
get-pixel-size
Gets the pixel size used for named icons.
method get-pixel-size (--> Int )
Return value; the pixel size used for named icons..
get-storage-type
Gets the type of representation being used by the Gnome::Gtk4::Image to store image data.
If the Gnome::Gtk4::Image has no image data, the return value will be GTK_IMAGE_EMPTY
.
method get-storage-type (--> GtkImageType )
Return value; image representation being used.
set-from-file
Sets a Gnome::Gtk4::Image to show a file.
See .new-from-file()
for details.
method set-from-file ( Str $filename )
$filename; a filename.
set-from-gicon
Sets a Gnome::Gtk4::Image to show a Gnome::Gio::R-Icon.
See .new-from-gicon()
for details.
method set-from-gicon ( N-Object() $icon )
$icon; an icon.
set-from-icon-name
Sets a Gnome::Gtk4::Image to show a named icon.
See .new-from-icon-name()
for details.
method set-from-icon-name ( Str $icon-name )
$icon-name; an icon name.
set-from-paintable
Sets a Gnome::Gtk4::Image to show a Gnome::Gdk4::R-Paintable.
See .new-from-paintable()
for details.
method set-from-paintable ( N-Object() $paintable )
$paintable; a Gnome::Gdk4::R-Paintable.
set-from-pixbuf
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.12
Sets a Gnome::Gtk4::Image to show a Gnome::GdkPixbuf::Pixbuf.
See .new-from-pixbuf()
for details.
Note: This is a helper for .set-from-paintable()
, and you can't get back the exact pixbuf once this is called, only a paintable.
method set-from-pixbuf ( N-Object() $pixbuf )
$pixbuf; a Gnome::GdkPixbuf::Pixbuf or undefined.
set-from-resource
Sets a Gnome::Gtk4::Image to show a resource.
See .new-from-resource()
for details.
method set-from-resource ( Str $resource-path )
$resource-path; a resource path.
set-icon-size
Suggests an icon size to the theme for named icons.
method set-icon-size ( GtkIconSize $icon-size )
$icon-size; the new icon size.
set-pixel-size
Sets the pixel size to use for named icons.
If the pixel size is set to a value != -1, it is used instead of the icon size set by .set-from-icon-name()
.
method set-pixel-size ( Int() $pixel-size )
$pixel-size; the new pixel size.