
Gnome::Gtk4::Picture
Description
The Gnome::Gtk4::Picture widget displays a Gnome::Gdk4::R-Paintable.
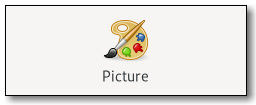
Many convenience functions are provided to make pictures simple to use. For example, if you want to load an image from a file, and then display it, there’s a convenience function to do this:
If the file isn’t loaded successfully, the picture will contain a “broken image” icon similar to that used in many web browsers. If you want to handle errors in loading the file yourself, for example by displaying an error message, then load the image with .new-from-file()
in class Gnome::Gdk4::Texture
, then create the Gnome::Gtk4::Picture with .new-for-paintable()
.
Sometimes an application will want to avoid depending on external data files, such as image files. See the documentation of Gnome::Gio::N-Resource for details. In this case, .new-for-resource()
and .set-resource()
should be used.
Gnome::Gtk4::Picture displays an image at its natural size. See Gnome::Gtk4::Image if you want to display a fixed-size image, such as an icon.
Sizing the paintable
You can influence how the paintable is displayed inside the Gnome::Gtk4::Picture by changing content-fit. See enumeration ContentFit
from Gnome::Gtk4::T-enums
for details. can-shrink can be unset to make sure that paintables are never made smaller than their ideal size - but be careful if you do not know the size of the paintable in use (like when displaying user-loaded images). This can easily cause the picture to grow larger than the screen. And halign defined in Gnome::Gtk4::Widget and valign defined in Gnome::Gtk4::Widget can be used to make sure the paintable doesn't fill all available space but is instead displayed at its original size.
CSS nodes
Gnome::Gtk4::Picture has a single CSS node with the name picture.
Accessibility
Gnome::Gtk4::Picture uses the GTK_ACCESSIBLE_ROLE_IMG role.
Uml Diagram
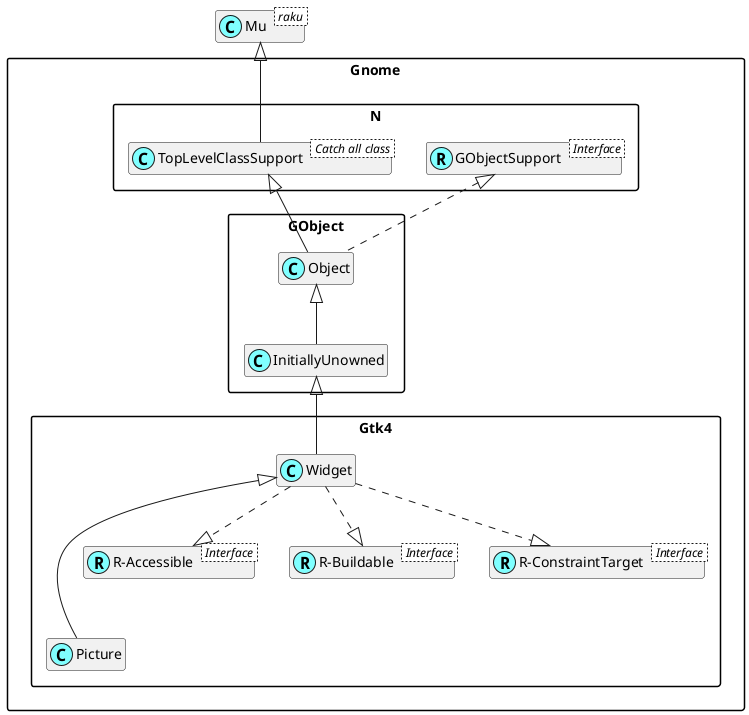
Class initialization
new
:native-object
Create an object using a native object from an object of the same type found elsewhere. See also Gnome::N::TopLevelSupportClass.
multi method new ( N-Object() :$native-object! )
new-picture
Creates a new empty Gnome::Gtk4::Picture widget.
method new-picture ( --> Gnome::Gtk4::Picture )
new-for-file
Creates a new Gnome::Gtk4::Picture displaying the given $file
.
If the file isn’t found or can’t be loaded, the resulting Gnome::Gtk4::Picture is empty.
If you need to detect failures to load the file, use .new-from-file()
in class Gnome::Gdk4::Texture
to load the file yourself, then create the Gnome::Gtk4::Picture from the texture.
method new-for-file ( N-Object() $file --> Gnome::Gtk4::Picture )
$file; a Gnome::Gio::R-File.
new-for-filename
Creates a new Gnome::Gtk4::Picture displaying the file $filename
.
This is a utility function that calls .new-for-file()
. See that function for details.
method new-for-filename ( Str $filename --> Gnome::Gtk4::Picture )
$filename; a filename.
new-for-paintable
Creates a new Gnome::Gtk4::Picture displaying $paintable
.
The Gnome::Gtk4::Picture will track changes to the $paintable
and update its size and contents in response to it.
method new-for-paintable ( N-Object() $paintable --> Gnome::Gtk4::Picture )
$paintable; a Gnome::Gdk4::R-Paintable.
new-for-pixbuf
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.12
Creates a new Gnome::Gtk4::Picture displaying $pixbuf
.
This is a utility function that calls .new-for-paintable()
, See that function for details.
The pixbuf must not be modified after passing it to this function.
method new-for-pixbuf ( N-Object() $pixbuf --> Gnome::Gtk4::Picture )
$pixbuf; a Gnome::GdkPixbuf::Pixbuf.
new-for-resource
Creates a new Gnome::Gtk4::Picture displaying the resource at $resource-path
.
This is a utility function that calls .new-for-file()
. See that function for details.
method new-for-resource ( Str $resource-path --> Gnome::Gtk4::Picture )
$resource-path; resource path to play back.
Methods
get-alternative-text
Gets the alternative textual description of the picture.
The returned string will be undefined if the picture cannot be described textually.
method get-alternative-text (--> Str )
Return value; the alternative textual description of $self
..
get-can-shrink
Returns whether the Gnome::Gtk4::Picture respects its contents size.
method get-can-shrink (--> Bool )
Return value; True
if the picture can be made smaller than its contents.
get-content-fit
Returns the fit mode for the content of the Gnome::Gtk4::Picture.
See enumeration ContentFit
from Gnome::Gtk4::T-enums
for details.
method get-content-fit (--> GtkContentFit )
Return value; the content fit mode.
get-file
Gets the Gnome::Gio::R-File currently displayed if $self
is displaying a file.
If $self
is not displaying a file, for example when .set-paintable()
was used, then undefined is returned.
method get-file (--> N-Object )
Return value; The Gnome::Gio::R-File displayed by $self
..
get-keep-aspect-ratio
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.8
Returns whether the Gnome::Gtk4::Picture preserves its contents aspect ratio.
method get-keep-aspect-ratio (--> Bool )
Return value; True
if the self tries to keep the contents' aspect ratio.
get-paintable
Gets the Gnome::Gdk4::R-Paintable being displayed by the Gnome::Gtk4::Picture.
method get-paintable (--> N-Object )
Return value; the displayed paintable.
set-alternative-text
Sets an alternative textual description for the picture contents.
It is equivalent to the "alt" attribute for images on websites.
This text will be made available to accessibility tools.
If the picture cannot be described textually, set this property to undefined.
method set-alternative-text ( Str $alternative-text )
$alternative-text; a textual description of the contents.
set-can-shrink
If set to True
, the $self
can be made smaller than its contents.
The contents will then be scaled down when rendering.
If you want to still force a minimum size manually, consider using .set-size-request()
in class Gnome::Gtk4::Widget
.
Also of note is that a similar function for growing does not exist because the grow behavior can be controlled via .set-halign()
in class Gnome::Gtk4::Widget
and .set-valign()
in class Gnome::Gtk4::Widget
.
method set-can-shrink ( Bool() $can-shrink )
$can-shrink; if
$self
can be made smaller than its contents.
set-content-fit
Sets how the content should be resized to fit the Gnome::Gtk4::Picture.
See enumeration ContentFit
from Gnome::Gtk4::T-enums
for details.
method set-content-fit ( GtkContentFit $content-fit )
$content-fit; the content fit mode.
set-file
Makes $self
load and display $file
.
See .new-for-file()
for details.
method set-file ( N-Object() $file )
$file; a Gnome::Gio::R-File.
set-filename
Makes $self
load and display the given $filename
.
This is a utility function that calls .set-file()
.
method set-filename ( Str $filename )
$filename; the filename to play.
set-keep-aspect-ratio
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.8
If set to True
, the $self
will render its contents according to their aspect ratio.
That means that empty space may show up at the top/bottom or left/right of $self
.
If set to False
or if the contents provide no aspect ratio, the contents will be stretched over the picture's whole area.
method set-keep-aspect-ratio ( Bool() $keep-aspect-ratio )
$keep-aspect-ratio; whether to keep aspect ratio.
set-paintable
Makes $self
display the given $paintable
.
If $paintable
is undefined, nothing will be displayed.
See .new-for-paintable()
for details.
method set-paintable ( N-Object() $paintable )
$paintable; a Gnome::Gdk4::R-Paintable.
set-pixbuf
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.12
Sets a Gnome::Gtk4::Picture to show a Gnome::GdkPixbuf::Pixbuf.
See .new-for-pixbuf()
for details.
This is a utility function that calls .set-paintable()
.
method set-pixbuf ( N-Object() $pixbuf )
$pixbuf; a Gnome::GdkPixbuf::Pixbuf.
set-resource
Makes $self
load and display the resource at the given $resource-path
.
This is a utility function that calls .set-file()
.
method set-resource ( Str $resource-path )
$resource-path; the resource to set.