
Gnome::Gtk4::TreeStore
Description
A tree-like data structure that can be used with the Gnome::Gtk4::TreeView.
The Gnome::Gtk4::TreeStore object is a list model for use with a Gnome::Gtk4::TreeView widget. It implements the Gnome::Gtk4::R-TreeModel interface, and consequently, can use all of the methods available there. It also implements the Gnome::Gtk4::R-TreeSortable interface so it can be sorted by the view. Finally, it also implements the tree [drag]Gnome::Gtk4::R-TreeDragSource and [drop]Gnome::Gtk4::R-TreeDragDest interfaces.
Gnome::Gtk4::TreeStore is deprecated since GTK 4.10, and should not be used in newly written code. You should use Gnome::Gtk4::TreeListModel for a tree-like model object.
GtkTreeStore as GtkBuildable
The GtkTreeStore implementation of the Gnome::Gtk4::R-Buildable interface allows to specify the model columns with a `<columns>` element that may contain multiple `<column>` elements, each specifying one model column. The “type” attribute specifies the data type for the column.
An example of a UI Definition fragment for a tree store:
Uml Diagram
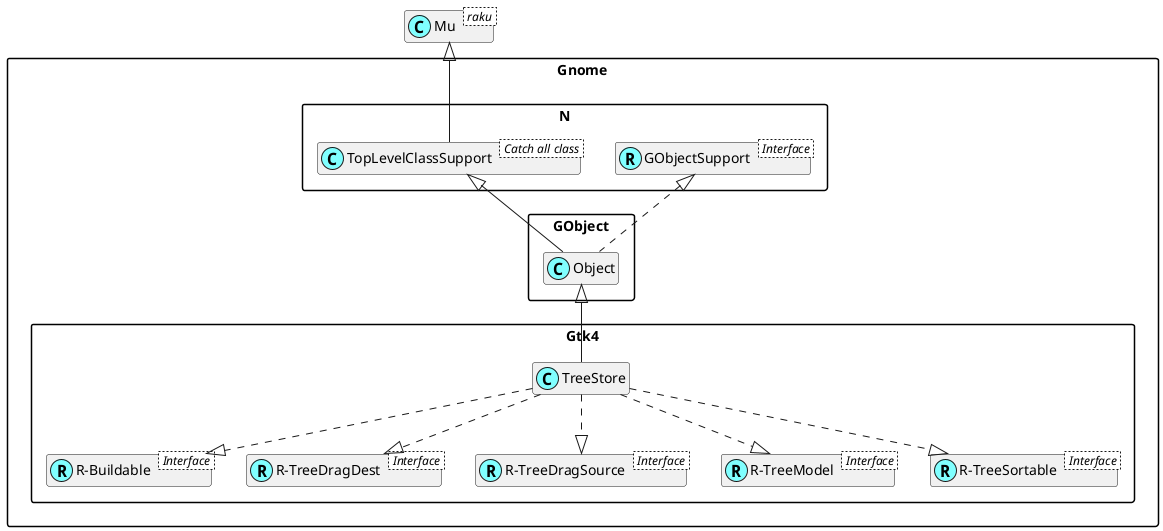
Class initialization
Note: The native version of this class is deprecated in gtk4-lib() since version 4.10
new
:native-object
Create an object using a native object from an object of the same type found elsewhere. See also Gnome::N::TopLevelSupportClass.
multi method new ( N-Object() :$native-object! )
new-treestore This function is not yet available
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Creates a new tree store.
The tree store will have $n-columns
, with each column using the corresponding type passed to this function.
Note that only types derived from standard GObject fundamental types are supported.
As an example:
will create a new Gnome::Gtk4::TreeStore with three columns of type int, gchararray, and Gnome::Gdk4::Texture respectively.
method new-treestore ( Int() $n-columns, … --> Gnome::Gtk4::TreeStore )
$n-columns; number of columns in the tree store.
…; …. Note that each argument must be specified as a type followed by its value!
newv This function is not yet available
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Creates a new tree store.
This constructor is meant for language bindings.
method newv ( Int() $n-columns, … --> Gnome::Gtk4::TreeStore )
$n-columns; number of columns in the tree store.
types; an array of Gnome::GObject::TreeStore types for the columns, from first to last. Note that each argument must be specified as a type followed by its value!
Methods
append
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Appends a new row to $tree-store
.
If $parent
is non-undefined, then it will append the new row after the last child of $parent
, otherwise it will append a row to the top level.
The $iter
parameter will be changed to point to this new row. The row will be empty after this function is called. To fill in values, you need to call .set()
or .set-value()
.
method append ( N-Object $iter, N-Object $parent )
$iter; An unset Gnome::Gtk4::N-TreeIter to set to the appended row
$parent; A valid Gnome::Gtk4::N-TreeIter
clear
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Removes all rows from $tree-store
method clear ( )
insert
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Creates a new row at $position
.
If parent is non-undefined, then the row will be made a child of $parent
. Otherwise, the row will be created at the toplevel.
If $position
is `-1` or is larger than the number of rows at that level, then the new row will be inserted to the end of the list.
The $iter
parameter will be changed to point to this new row. The row will be empty after this function is called. To fill in values, you need to call .set()
or .set-value()
.
method insert ( N-Object $iter, N-Object $parent, Int() $position )
$iter; An unset Gnome::Gtk4::N-TreeIter to set to the new row
$parent; A valid Gnome::Gtk4::N-TreeIter
$position; position to insert the new row, or -1 for last.
insert-after
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Inserts a new row after $sibling
.
If $sibling
is undefined, then the row will be prepended to $parent
’s children.
If $parent
and $sibling
are undefined, then the row will be prepended to the toplevel.
If both $sibling
and $parent
are set, then $parent
must be the parent of $sibling
. When $sibling
is set, $parent
is optional.
The $iter
parameter will be changed to point to this new row. The row will be empty after this function is called. To fill in values, you need to call .set()
or .set-value()
.
method insert-after ( N-Object $iter, N-Object $parent, N-Object $sibling )
$iter; An unset Gnome::Gtk4::N-TreeIter to set to the new row
$parent; A valid Gnome::Gtk4::N-TreeIter
$sibling; A valid Gnome::Gtk4::N-TreeIter
insert-before
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Inserts a new row before $sibling
.
If $sibling
is undefined, then the row will be appended to $parent
’s children.
If $parent
and $sibling
are undefined, then the row will be appended to the toplevel.
If both $sibling
and $parent
are set, then $parent
must be the parent of $sibling
. When $sibling
is set, $parent
is optional.
The $iter
parameter will be changed to point to this new row. The row will be empty after this function is called. To fill in values, you need to call .set()
or .set-value()
.
method insert-before ( N-Object $iter, N-Object $parent, N-Object $sibling )
$iter; An unset Gnome::Gtk4::N-TreeIter to set to the new row
$parent; A valid Gnome::Gtk4::N-TreeIter
$sibling; A valid Gnome::Gtk4::N-TreeIter
insert-with-values This function is not yet available
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Creates a new row at the given $position
.
The $iter
parameter will be changed to point to this new row.
If $position
is -1, or larger than the number of rows on the list, then the new row will be appended to the list. The row will be filled with the values given to this function.
Calling
gtk_tree_store_insert_with_values (tree_store, iter, position, ...)
has the same effect as calling
with the different that the former will only emit a row_inserted signal, while the latter will emit row_inserted, row_changed and if the tree store is sorted, rows_reordered.
Since emitting the rows_reordered signal repeatedly can affect the performance of the program, .insert-with-values()
should generally be preferred when inserting rows in a sorted tree store.
method insert-with-values ( N-Object $iter, N-Object $parent, Int() $position, … )
$iter; An unset Gnome::Gtk4::N-TreeIter to set the new row
$parent; A valid Gnome::Gtk4::N-TreeIter
$position; position to insert the new row, or -1 to append after existing rows.
…; …. Note that each argument must be specified as a type followed by its value!
insert-with-valuesv
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
A variant of .insert-with-values()
which takes the columns and values as two arrays, instead of varargs.
This function is mainly intended for language bindings.
method insert-with-valuesv ( N-Object $iter, N-Object $parent, Int() $position, Array[Int] $columns, N-Object $values, Int() $n-values )
$iter; An unset Gnome::Gtk4::N-TreeIter to set the new row
$parent; A valid Gnome::Gtk4::N-TreeIter
$position; position to insert the new row, or -1 for last.
$columns; an array of column numbers.
$values; an array of GValues
$n-values; the length of the
$columns
and$values
arrays.
is-ancestor
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Checks if $iter
is an ancestor of $descendant
.
method is-ancestor ( N-Object $iter, N-Object $descendant --> Bool )
$iter; A valid Gnome::Gtk4::N-TreeIter
$descendant; A valid Gnome::Gtk4::N-TreeIter
Return value; true if $iter
is an ancestor of $descendant
, and false otherwise.
iter-depth
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Returns the depth of the position pointed by the iterator
The depth will be 0 for anything on the root level, 1 for anything down a level, etc.
method iter-depth ( N-Object $iter --> Int )
$iter; A valid Gnome::Gtk4::N-TreeIter
Return value; The depth of the position pointed by the iterator.
iter-is-valid
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Checks if the given iter is a valid iter for this Gnome::Gtk4::TreeStore.
This function is slow. Only use it for debugging and/or testing purposes.
method iter-is-valid ( N-Object $iter --> Bool )
$iter; the iterator to check
Return value; true if the iter is valid, and false otherwise.
move-after
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Moves $iter
in $tree-store
to the position after $position
. $iter
and $position
should be in the same level.
Note that this function only works with unsorted stores.
If $position
is undefined, $iter
will be moved to the start of the level.
method move-after ( N-Object $iter, N-Object $position )
$iter; A Gnome::Gtk4::N-TreeIter.
$position; A Gnome::Gtk4::N-TreeIter.
move-before
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Moves $iter
in $tree-store
to the position before $position
. $iter
and $position
should be in the same level.
Note that this function only works with unsorted stores.
If $position
is undefined, $iter
will be moved to the end of the level.
method move-before ( N-Object $iter, N-Object $position )
$iter; A Gnome::Gtk4::N-TreeIter
$position; A Gnome::Gtk4::N-TreeIter
prepend
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Prepends a new row to $tree-store
.
If $parent
is non-undefined, then it will prepend the new row before the first child of $parent
, otherwise it will prepend a row to the top level. The iter parameter will be changed to point to this new row. The row will be empty after this function is called. To fill in values, you need to call .set()
or .set-value()
.
method prepend ( N-Object $iter, N-Object $parent )
$iter; An unset Gnome::Gtk4::N-TreeIter to set to the prepended row
$parent; A valid Gnome::Gtk4::N-TreeIter
remove
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Removes $iter
from $tree-store
.
After being removed, $iter
is set to the next valid row at that level, or invalidated if it previously pointed to the last one.
method remove ( N-Object $iter --> Bool )
$iter; A valid Gnome::Gtk4::N-TreeIter
Return value; true if $iter
is still valid, and false otherwise.
reorder
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Reorders the children of $parent
in $tree-store
to follow the order indicated by $new-order
.
Note that this function only works with unsorted stores.
method reorder ( N-Object $parent, Array[Int] $new-order )
$parent; the parent of the children to re-order
$new-order; an array of integers mapping the new position of each child to its old position before the re-ordering, i.e. new_order[newpos] = oldpos`.
set This function is not yet available
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Sets the value of one or more cells in the row referenced by $iter
.
The variable argument list should contain integer column numbers, each column number followed by the value to be set.
The list is terminated by a value of `-1`.
For example, to set column 0 with type G_TYPE_STRING to “Foo”, you would write
The value will be referenced by the store if it is a G_TYPE_OBJECT, and it will be copied if it is a G_TYPE_STRING or G_TYPE_BOXED.
method set ( N-Object $iter, … )
$iter; A valid Gnome::Gtk4::N-TreeIter for the row being modified
…; …. Note that each argument must be specified as a type followed by its value!
set-column-types This function is not yet available
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Sets the type of the columns in a tree store.
This function is meant primarily for types that inherit from Gnome::Gtk4::TreeStore, and should only be used when constructing a new Gnome::Gtk4::TreeStore.
This functions cannot be called after a row has been added, or a method on the Gnome::Gtk4::R-TreeModel interface is called on the tree store.
method set-column-types ( Int() $n-columns, … )
$n-columns; Number of columns for the tree store.
types; An array of Gnome::GObject::TreeStore types, one for each column. Note that each argument must be specified as a type followed by its value!
set-valist This function is not yet available
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
A version of .set()
using va_list.
method set-valist ( N-Object $iter, … )
$iter; A valid Gnome::Gtk4::N-TreeIter for the row being modified
var-args; va_list of column/value pairs. Note that each argument must be specified as a type followed by its value!
set-value
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Sets the data in the cell specified by $iter
and $column
.
The type of $value
must be convertible to the type of the column.
method set-value ( N-Object $iter, Int() $column, N-Object $value )
$iter; A valid Gnome::Gtk4::N-TreeIter for the row being modified
$column; column number to modify.
$value; new value for the cell
set-valuesv
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
A variant of .set-valist()
which takes the columns and values as two arrays, instead of using variadic arguments.
This function is mainly intended for language bindings or in case the number of columns to change is not known until run-time.
method set-valuesv ( N-Object $iter, Array[Int] $columns, N-Object $values, Int() $n-values )
$iter; A valid Gnome::Gtk4::N-TreeIter for the row being modified
$columns; an array of column numbers.
$values; an array of GValues
$n-values; the length of the
$columns
and$values
arrays.
swap
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Swaps $a
and $b
in the same level of $tree-store
.
Note that this function only works with unsorted stores.
method swap ( N-Object $a, N-Object $b )
$a; A Gnome::Gtk4::N-TreeIter.
$b; Another Gnome::Gtk4::N-TreeIter.