
Gnome::Gtk4::ListView
Description
Gnome::Gtk4::ListView presents a large dynamic list of items.
Gnome::Gtk4::ListView uses its factory to generate one row widget for each visible item and shows them in a linear display, either vertically or horizontally.
The show-separators property offers a simple way to display separators between the rows.
Gnome::Gtk4::ListView allows the user to select items according to the selection characteristics of the model. For models that allow multiple selected items, it is possible to turn on _rubberband selection_, using enable-rubberband.
If you need multiple columns with headers, see Gnome::Gtk4::ColumnView.
To learn more about the list widget framework, see the [overview](section-list-widget.html).
An example of using Gnome::Gtk4::ListView:
CSS nodes
Gnome::Gtk4::ListView uses a single CSS node named listview. It may carry the `.separators` style class, when show-separators property is set. Each child widget uses a single CSS node named row. If the activatable defined in Gnome::Gtk4::ListItem property is set, the corresponding row will have the `.activatable` style class. For rubberband selection, a node with name rubberband is used.
The main listview node may also carry style classes to select the style of [list presentation](ListContainers.html#list-styles): .rich-list, .navigation-sidebar or .data-table.
Accessibility
Gnome::Gtk4::ListView uses the GTK_ACCESSIBLE_ROLE_LIST
role, and the list items use the GTK_ACCESSIBLE_ROLE_LIST_ITEM
role.
Uml Diagram
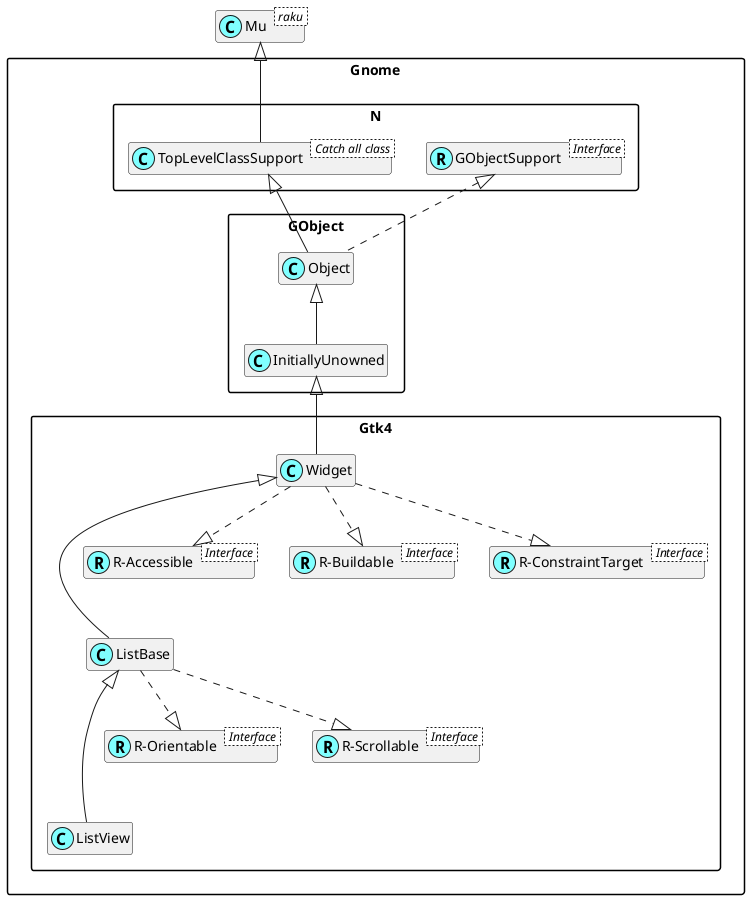
Class initialization
new
:native-object
Create an object using a native object from an object of the same type found elsewhere. See also Gnome::N::TopLevelSupportClass.
multi method new ( N-Object() :$native-object! )
new-listview
Creates a new Gnome::Gtk4::ListView that uses the given $factory
for mapping items to widgets.
The function takes ownership of the arguments, so you can write code like
method new-listview ( N-Object() $model, N-Object() $factory --> Gnome::Gtk4::ListView )
$model; (transfer ownership: full) the model to use.
$factory; (transfer ownership: full) The factory to populate items with.
Methods
get-enable-rubberband
Returns whether rows can be selected by dragging with the mouse.
method get-enable-rubberband (--> Bool )
Return value; True
if rubberband selection is enabled.
get-factory
Gets the factory that's currently used to populate list items.
method get-factory (--> N-Object )
Return value; The factory in use.
get-header-factory
Gets the factory that's currently used to populate section headers.
method get-header-factory (--> N-Object )
Return value; The factory in use.
get-model
Gets the model that's currently used to read the items displayed.
method get-model (--> N-Object )
Return value; The model in use.
get-show-separators
Returns whether the list box should show separators between rows.
method get-show-separators (--> Bool )
Return value; True
if the list box shows separators.
get-single-click-activate
Returns whether rows will be activated on single click and selected on hover.
method get-single-click-activate (--> Bool )
Return value; True
if rows are activated on single click.
get-tab-behavior
Gets the behavior set for the <kbd>Tab</kbd> key.
method get-tab-behavior (--> GtkListTabBehavior )
Return value; The behavior of the <kbd>Tab</kbd> key.
scroll-to
Scrolls to the item at the given position and performs the actions specified in $flags
.
This function works no matter if the listview is shown or focused. If it isn't, then the changes will take effect once that happens.
method scroll-to ( UInt() $pos, UInt $flags, N-Object $scroll )
$pos; position of the item. Must be less than the number of items in the view..
$flags; actions to perform.
$scroll; (transfer ownership: full) details of how to perform the scroll operation or undefined to scroll into view
set-enable-rubberband
Sets whether selections can be changed by dragging with the mouse.
method set-enable-rubberband ( Bool() $enable-rubberband )
$enable-rubberband;
True
to enable rubberband selection.
set-factory
Sets the Gnome::Gtk4::ListItemFactory to use for populating list items.
method set-factory ( N-Object() $factory )
$factory; the factory to use.
set-header-factory
Sets the Gnome::Gtk4::ListItemFactory to use for populating the Gnome::Gtk4::ListHeader objects used in section headers.
If this factory is set to undefined, the list will not show section headers.
method set-header-factory ( N-Object() $factory )
$factory; the factory to use.
set-model
Sets the model to use.
This must be a Gnome::Gtk4::R-SelectionModel to use.
method set-model ( N-Object() $model )
$model; the model to use.
set-show-separators
Sets whether the list box should show separators between rows.
method set-show-separators ( Bool() $show-separators )
$show-separators;
True
to show separators.
set-single-click-activate
Sets whether rows should be activated on single click and selected on hover.
method set-single-click-activate ( Bool() $single-click-activate )
$single-click-activate;
True
to activate items on single click.
set-tab-behavior
Sets the behavior of the <kbd>Tab</kbd> and <kbd>Shift</kbd>+<kbd>Tab</kbd> keys.
method set-tab-behavior ( GtkListTabBehavior $tab-behavior )
$tab-behavior; The desired tab behavior.
Signals
activate
Emitted when a row has been activated by the user, usually via activating the GtkListView|list.activate-item action.
This allows for a convenient way to handle activation in a listview. See .set-activatable()
in class Gnome::Gtk4::ListItem
for details on how to use this signal.
method handler ( guint $position, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::ListView :$_widget, *C<user>-options )
$position; position of item to activate.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::ListView object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.