
Gnome::Gtk4::FileChooserDialog
Description
Gnome::Gtk4::FileChooserDialog is a dialog suitable for use with “File Open” or “File Save” commands.
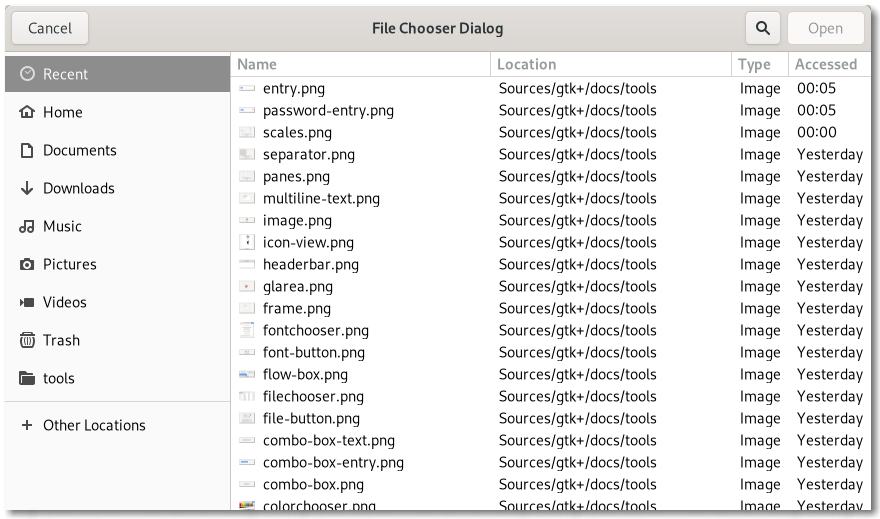
This widget works by putting a Gnome::Gtk4::FileChooserWidget inside a Gnome::Gtk4::Dialog. It exposes the Gnome::Gtk4::R-FileChooser interface, so you can use all of the Gnome::Gtk4::R-FileChooser functions on the file chooser dialog as well as those for Gnome::Gtk4::Dialog.
Note that Gnome::Gtk4::FileChooserDialog does not have any methods of its own. Instead, you should use the functions that work on a Gnome::Gtk4::R-FileChooser.
If you want to integrate well with the platform you should use the Gnome::Gtk4::FileChooserNative API, which will use a platform-specific dialog if available and fall back to Gnome::Gtk4::FileChooserDialog otherwise.
Typical usage
In the simplest of cases, you can the following code to use Gnome::Gtk4::FileChooserDialog to select a file for opening:
To use a dialog for saving, you can use this:
Setting up a file chooser dialog
There are various cases in which you may need to use a Gnome::Gtk4::FileChooserDialog:
To select a file for opening, use
GTK_FILE_CHOOSER_ACTION_OPEN
.To save a file for the first time, use
GTK_FILE_CHOOSER_ACTION_SAVE
, and suggest a name such as “Untitled” with.set-current-name()
in classGnome::Gtk4::R-FileChooser
.To save a file under a different name, use
GTK_FILE_CHOOSER_ACTION_SAVE
, and set the existing file with.set-file()
in classGnome::Gtk4::R-FileChooser
.To choose a folder instead of a filem use
GTK_FILE_CHOOSER_ACTION_SELECT_FOLDER
.
In general, you should only cause the file chooser to show a specific folder when it is appropriate to use .set-file()
in class Gnome::Gtk4::R-FileChooser
, i.e. when you are doing a “Save As” command and you already have a file saved somewhere.
Response Codes
Gnome::Gtk4::FileChooserDialog inherits from Gnome::Gtk4::Dialog, so buttons that go in its action area have response codes such as GTK_RESPONSE_ACCEPT
and GTK_RESPONSE_CANCEL
. For example, you could call .newfilechooserdialog()
as follows:
This will create buttons for “Cancel” and “Open” that use predefined response identifiers from enumeration ResponseType
from Gnome::Gtk4::T-dialog
. For most dialog boxes you can use your own custom response codes rather than the ones in enumeration ResponseType
from Gnome::Gtk4::T-dialog
, but Gnome::Gtk4::FileChooserDialog assumes that its “accept”-type action, e.g. an “Open” or “Save” button, will have one of the following response codes:
GTK_RESPONSE_ACCEPT
GTK_RESPONSE_OK
GTK_RESPONSE_YES
GTK_RESPONSE_APPLY
This is because Gnome::Gtk4::FileChooserDialog must intercept responses and switch to folders if appropriate, rather than letting the dialog terminate — the implementation uses these known response codes to know which responses can be blocked if appropriate.
To summarize, make sure you use a predefined response code when you use Gnome::Gtk4::FileChooserDialog to ensure proper operation.
CSS nodes
Gnome::Gtk4::FileChooserDialog has a single CSS node with the name window and style class `.filechooser`.
Uml Diagram
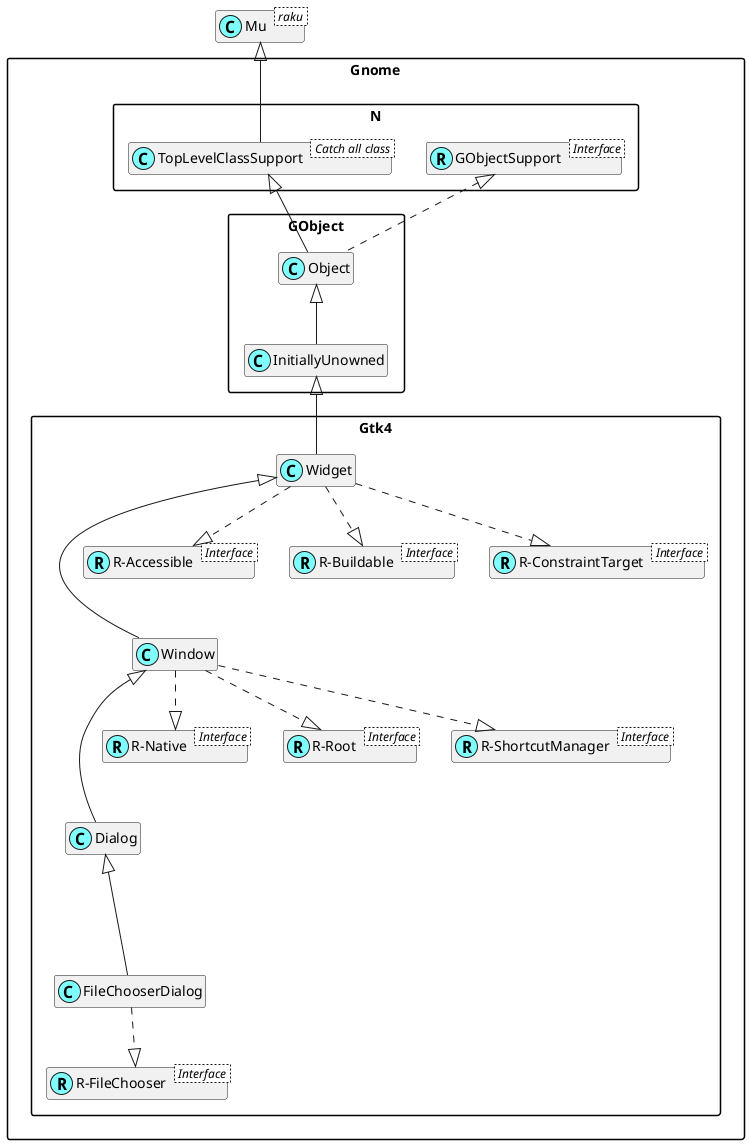
Class initialization
Note: The native version of this class is deprecated in gtk4-lib() since version 4.10
new
:native-object
Create an object using a native object from an object of the same type found elsewhere. See also Gnome::N::TopLevelSupportClass.
multi method new ( N-Object() :$native-object! )
new-filechooserdialog This function is not yet available
Note: The native version of this routine is deprecated in gtk4-lib() since version 4.10
Creates a new Gnome::Gtk4::FileChooserDialog.
This function is analogous to .new-with-buttons()
in class Gnome::Gtk4::Dialog
.
method new-filechooserdialog ( Str $title, N-Object() $parent, GtkFileChooserAction $action, Str $first-button-text, … --> Gnome::Gtk4::FileChooserDialog )
$title; Title of the dialog.
$parent; Transient parent of the dialog.
$action; Open or save mode for the dialog.
$first-button-text; text to go in the first button.
…; …. Note that each argument must be specified as a type followed by its value!