
Gnome::Gtk4::PrintOperation
Description
Gnome::Gtk4::PrintOperation is the high-level, portable printing API.
It looks a bit different than other GTK dialogs such as the Gnome::Gtk4::R-FileChooser, since some platforms don’t expose enough infrastructure to implement a good print dialog. On such platforms, Gnome::Gtk4::PrintOperation uses the native print dialog. On platforms which do not provide a native print dialog, GTK uses its own, see Gnome::Gtk4::PrintUnixDialog.
The typical way to use the high-level printing API is to create a Gnome::Gtk4::PrintOperation object with .newprintoperation()
when the user selects to print. Then you set some properties on it, e.g. the page size, any Gnome::Gtk4::PrintSettings from previous print operations, the number of pages, the current page, etc.
Then you start the print operation by calling .run()
]. It will then show a dialog, let the user select a printer and options. When the user finished the dialog, various signals will be emitted on the Gnome::Gtk4::PrintOperation, the main one being draw-page, which you are supposed to handle and render the page on the provided Gnome::Gtk4::PrintContext using Cairo.
The high-level printing API
By default Gnome::Gtk4::PrintOperation uses an external application to do print preview. To implement a custom print preview, an application must connect to the preview signal. The functions .render-page()
in class Gnome::Gtk4::R-PrintOperationPreview], .end-preview()
in class Gnome::Gtk4::R-PrintOperationPreview] and .is-selected()
in class Gnome::Gtk4::R-PrintOperationPreview] are useful when implementing a print preview.
Uml Diagram
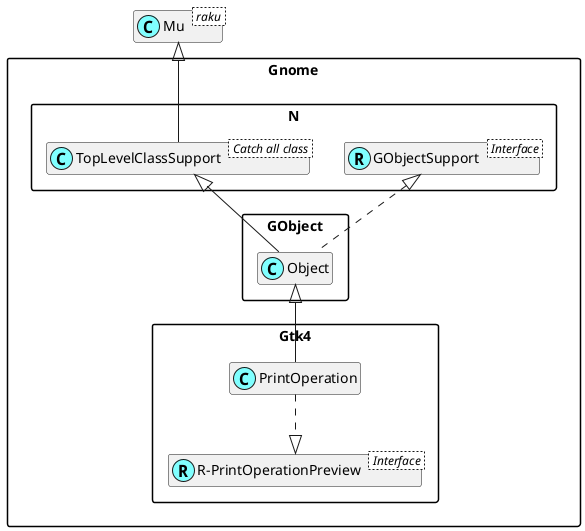
Class initialization
new
:native-object
Create an object using a native object from an object of the same type found elsewhere. See also Gnome::N::TopLevelSupportClass.
multi method new ( N-Object() :$native-object! )
new-printoperation
Creates a new Gnome::Gtk4::PrintOperation.
method new-printoperation ( --> Gnome::Gtk4::PrintOperation )
Methods
cancel
Cancels a running print operation.
This function may be called from a begin-print, paginate or draw-page signal handler to stop the currently running print operation.
method cancel ( )
draw-page-finish
Signal that drawing of particular page is complete.
It is called after completion of page drawing (e.g. drawing in another thread). If .set-defer-drawing()
] was called before, then this function has to be called by application. Otherwise it is called by GTK itself.
method draw-page-finish ( )
get-default-page-setup
Returns the default page setup.
method get-default-page-setup (--> N-Object )
Return value; the default page setup.
get-embed-page-setup
Gets whether page setup selection combos are embedded
method get-embed-page-setup (--> Bool )
Return value; whether page setup selection combos are embedded.
get-error
Call this when the result of a print operation is GTK_PRINT_OPERATION_RESULT_ERROR
.
It can be called either after .run()
] returns, or in the done signal handler.
The returned Gnome::Glib::N-Error will contain more details on what went wrong.
method get-error ( CArray[N-Error] $err )
$err; Error object. When defined, an error can be returned when there is one. Use
Pointer
when you want to ignore the error. .
get-has-selection
Gets whether there is a selection.
method get-has-selection (--> Bool )
Return value; whether there is a selection.
get-n-pages-to-print
Returns the number of pages that will be printed.
Note that this value is set during print preparation phase (GTK_PRINT_STATUS_PREPARING
), so this function should never be called before the data generation phase (GTK_PRINT_STATUS_GENERATING_DATA
). You can connect to the status-changed signal and call .get-n-pages-to-print()
when print status is GTK_PRINT_STATUS_GENERATING_DATA
.
This is typically used to track the progress of print operation.
method get-n-pages-to-print (--> Int )
Return value; the number of pages that will be printed.
get-print-settings
Returns the current print settings.
Note that the return value is undefined until either .set-print-settings()
] or .run()
] have been called.
method get-print-settings (--> N-Object )
Return value; the current print settings of $op
..
get-status
Returns the status of the print operation.
Also see .get-status-string()
].
method get-status (--> GtkPrintStatus )
Return value; the status of the print operation.
get-status-string
Returns a string representation of the status of the print operation.
The string is translated and suitable for displaying the print status e.g. in a Gnome::Gtk4::Statusbar.
Use .get-status()
] to obtain a status value that is suitable for programmatic use.
method get-status-string (--> Str )
Return value; a string representation of the status of the print operation.
get-support-selection
Gets whether the application supports print of selection
method get-support-selection (--> Bool )
Return value; whether the application supports print of selection.
is-finished
A convenience function to find out if the print operation is finished.
a print operation is finished if its status is either GTK_PRINT_STATUS_FINISHED
or GTK_PRINT_STATUS_FINISHED_ABORTED
.
Note: when you enable print status tracking the print operation can be in a non-finished state even after done has been called, as the operation status then tracks the print job status on the printer.
method is-finished (--> Bool )
Return value; True
, if the print operation is finished..
run
Runs the print operation.
Normally that this function does not return until the rendering of all pages is complete. You can connect to the status-changed signal on $op
to obtain some information about the progress of the print operation.
Furthermore, it may use a recursive mainloop to show the print dialog.
If you set the [Gtk.PrintOperation:allow-async] property, the operation will run asynchronously if this is supported on the platform. The done signal will be emitted with the result of the operation when the it is done (i.e. when the dialog is canceled, or when the print succeeds or fails).
Note that .run()
can only be called once on a given Gnome::Gtk4::PrintOperation.
method run ( GtkPrintOperationAction $action, N-Object() $parent, CArray[N-Error] $err --> GtkPrintOperationResult )
$action; the action to start.
$parent; Transient parent of the dialog.
$err; Error object. When defined, an error can be returned when there is one. Use
Pointer
when you want to ignore the error. .
Return value; the result of the print operation. A return value of GTK_PRINT_OPERATION_RESULT_APPLY
indicates that the printing was completed successfully. In this case, it is a good idea to obtain the used print settings with .get-print-settings()
] and store them for reuse with the next print operation. A value of GTK_PRINT_OPERATION_RESULT_IN_PROGRESS
means the operation is running asynchronously, and will emit the done signal when done..
set-allow-async
Sets whether .run()
may return before the print operation is completed.
Note that some platforms may not allow asynchronous operation.
method set-allow-async ( Bool() $allow-async )
$allow-async;
True
to allow asynchronous operation.
set-current-page
Sets the current page.
If this is called before .run()
], the user will be able to select to print only the current page.
Note that this only makes sense for pre-paginated documents.
method set-current-page ( Int() $current-page )
$current-page; the current page, 0-based.
set-custom-tab-label
Sets the label for the tab holding custom widgets.
method set-custom-tab-label ( Str $label )
$label; the label to use, or undefined to use the default label.
set-default-page-setup
Makes $default-page-setup
the default page setup for $op
.
This page setup will be used by .run()
], but it can be overridden on a per-page basis by connecting to the request-page-setup signal.
method set-default-page-setup ( N-Object() $default-page-setup )
$default-page-setup; a Gnome::Gtk4::PageSetup.
set-defer-drawing
Sets up the Gnome::Gtk4::PrintOperation to wait for calling of .draw-page-finish()
from application.
This can be used for drawing page in another thread.
This function must be called in the callback of the draw-page signal.
method set-defer-drawing ( )
set-embed-page-setup
Embed page size combo box and orientation combo box into page setup page.
Selected page setup is stored as default page setup in Gnome::Gtk4::PrintOperation.
method set-embed-page-setup ( Bool() $embed )
$embed;
True
to embed page setup selection in the Gnome::Gtk4::PrintUnixDialog.
set-export-filename
Sets up the Gnome::Gtk4::PrintOperation to generate a file instead of showing the print dialog.
The intended use of this function is for implementing “Export to PDF” actions. Currently, PDF is the only supported format.
“Print to PDF” support is independent of this and is done by letting the user pick the “Print to PDF” item from the list of printers in the print dialog.
method set-export-filename ( Str $filename )
$filename; the filename for the exported file.
set-has-selection
Sets whether there is a selection to print.
Application has to set number of pages to which the selection will draw by .set-n-pages()
] in a handler for the begin-print signal.
method set-has-selection ( Bool() $has-selection )
$has-selection;
True
indicates that a selection exists.
set-job-name
Sets the name of the print job.
The name is used to identify the job (e.g. in monitoring applications like eggcups).
If you don’t set a job name, GTK picks a default one by numbering successive print jobs.
method set-job-name ( Str $job-name )
$job-name; a string that identifies the print job.
set-n-pages
Sets the number of pages in the document.
This must be set to a positive number before the rendering starts. It may be set in a begin-print signal handler.
Note that the page numbers passed to the request-page-setup and draw-page signals are 0-based, i.e. if the user chooses to print all pages, the last draw-page signal will be for page $n-pages
- 1.
method set-n-pages ( Int() $n-pages )
$n-pages; the number of pages.
set-print-settings
Sets the print settings for $op
.
This is typically used to re-establish print settings from a previous print operation, see .run()
].
method set-print-settings ( N-Object() $print-settings )
$print-settings; Gnome::Gtk4::PrintSettings.
set-show-progress
If $show-progress
is True
, the print operation will show a progress dialog during the print operation.
method set-show-progress ( Bool() $show-progress )
$show-progress;
True
to show a progress dialog.
set-support-selection
Sets whether selection is supported by Gnome::Gtk4::PrintOperation.
method set-support-selection ( Bool() $support-selection )
$support-selection;
True
to support selection.
set-track-print-status
If track_status is True
, the print operation will try to continue report on the status of the print job in the printer queues and printer.
This can allow your application to show things like “out of paper” issues, and when the print job actually reaches the printer.
This function is often implemented using some form of polling, so it should not be enabled unless needed.
method set-track-print-status ( Bool() $track-status )
$track-status;
True
to track status after printing.
set-unit
Sets up the transformation for the cairo context obtained from Gnome::Gtk4::PrintContext in such a way that distances are measured in units of $unit
.
method set-unit ( GtkUnit $unit )
$unit; the unit to use.
set-use-full-page
If $full-page
is True
, the transformation for the cairo context obtained from Gnome::Gtk4::PrintContext puts the origin at the top left corner of the page.
This may not be the top left corner of the sheet, depending on page orientation and the number of pages per sheet). Otherwise, the origin is at the top left corner of the imageable area (i.e. inside the margins).
method set-use-full-page ( Bool() $full-page )
$full-page;
True
to set up the Gnome::Gtk4::PrintContext for the full page.
Signals
begin-print
Emitted after the user has finished changing print settings in the dialog, before the actual rendering starts.
A typical use for begin-print is to use the parameters from the Gnome::Gtk4::PrintContext and paginate the document accordingly, and then set the number of pages with .set-n-pages()
].
method handler ( N-Object $context, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::PrintOperation :$_widget, *C<user>-options )
$context; the Gnome::Gtk4::PrintContext for the current operation.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::PrintOperation object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
create-custom-widget
Emitted when displaying the print dialog.
If you return a widget in a handler for this signal it will be added to a custom tab in the print dialog. You typically return a container widget with multiple widgets in it.
The print dialog owns the returned widget, and its lifetime is not controlled by the application. However, the widget is guaranteed to stay around until the custom-widget-apply signal is emitted on the operation. Then you can read out any information you need from the widgets.
method handler ( Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::PrintOperation :$_widget, *C<user>-options )
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::PrintOperation object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
custom-widget-apply
Emitted right before begin-print if you added a custom widget in the create-custom-widget handler.
When you get this signal you should read the information from the custom widgets, as the widgets are not guaranteed to be around at a later time.
method handler ( N-Object $widget, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::PrintOperation :$_widget, *C<user>-options )
$widget; the custom widget added in create-custom-widget.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::PrintOperation object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
done
Emitted when the print operation run has finished doing everything required for printing. $result
gives you information about what happened during the run. If $result
is GTK_PRINT_OPERATION_RESULT_ERROR
then you can call .get-error()
] for more information.
If you enabled print status tracking then .is-finished()
] may still return False
after the done signal was emitted.
method handler ( $result, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::PrintOperation :$_widget, *C<user>-options )
$result; the result of the print operation.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::PrintOperation object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
draw-page
Emitted for every page that is printed.
The signal handler must render the $page-nr
's page onto the cairo context obtained from $context
using .get-cairo-context()
in class Gnome::Gtk4::PrintContext].
Use .set-use-full-page()
] and .set-unit()
] before starting the print operation to set up the transformation of the cairo context according to your needs.
method handler ( N-Object $context, gint $page-nr, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::PrintOperation :$_widget, *C<user>-options )
$context; the Gnome::Gtk4::PrintContext for the current operation.
$page-nr; the number of the currently printed page (0-based).
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::PrintOperation object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
end-print
Emitted after all pages have been rendered.
A handler for this signal can clean up any resources that have been allocated in the begin-print handler.
method handler ( N-Object $context, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::PrintOperation :$_widget, *C<user>-options )
$context; the Gnome::Gtk4::PrintContext for the current operation.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::PrintOperation object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
paginate
Emitted after the begin-print signal, but before the actual rendering starts.
It keeps getting emitted until a connected signal handler returns True
.
The paginate signal is intended to be used for paginating a document in small chunks, to avoid blocking the user interface for a long time. The signal handler should update the number of pages using .set-n-pages()
], and return True
if the document has been completely paginated.
If you don't need to do pagination in chunks, you can simply do it all in the begin-print handler, and set the number of pages from there.
method handler ( N-Object $context, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::PrintOperation :$_widget, *C<user>-options --> gboolean )
$context; the Gnome::Gtk4::PrintContext for the current operation.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::PrintOperation object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
Return value; True
if pagination is complete
preview
Gets emitted when a preview is requested from the native dialog.
The default handler for this signal uses an external viewer application to preview.
To implement a custom print preview, an application must return True
from its handler for this signal. In order to use the provided $context
for the preview implementation, it must be given a suitable cairo context with .set-cairo-context()
in class Gnome::Gtk4::PrintContext].
The custom preview implementation can use .is-selected()
in class Gnome::Gtk4::R-PrintOperationPreview] and .render-page()
in class Gnome::Gtk4::R-PrintOperationPreview] to find pages which are selected for print and render them. The preview must be finished by calling .end-preview()
in class Gnome::Gtk4::R-PrintOperationPreview] (typically in response to the user clicking a close button).
method handler ( N-Object $preview, N-Object $context, N-Object $parent, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::PrintOperation :$_widget, *C<user>-options --> gboolean )
$preview; the Gnome::Gtk4::R-PrintOperationPreview for the current operation.
$context; the Gnome::Gtk4::PrintContext that will be used.
$parent; the Gnome::Gtk4::Window to use as window parent.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::PrintOperation object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
Return value; True
if the listener wants to take over control of the preview
request-page-setup
Emitted once for every page that is printed.
This gives the application a chance to modify the page setup. Any changes done to $setup
will be in force only for printing this page.
method handler ( N-Object $context, gint $page-nr, N-Object $setup, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::PrintOperation :$_widget, *C<user>-options )
$context; the Gnome::Gtk4::PrintContext for the current operation.
$page-nr; the number of the currently printed page (0-based).
$setup; the Gnome::Gtk4::PageSetup.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::PrintOperation object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
status-changed
Emitted at between the various phases of the print operation.
See enumeration PrintStatus
from Gnome::Gtk4::T-printoperation
for the phases that are being discriminated. Use .get-status()
] to find out the current status.
method handler ( Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::PrintOperation :$_widget, *C<user>-options )
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::PrintOperation object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.
update-custom-widget
Emitted after change of selected printer.
The actual page setup and print settings are passed to the custom widget, which can actualize itself according to this change.
method handler ( N-Object $widget, N-Object $setup, N-Object $settings, Int :$_handle_id, N-GObject :$_native-object, Gnome::Gtk4::PrintOperation :$_widget, *C<user>-options )
$widget; the custom widget added in create-custom-widget.
$setup; actual page setup.
$settings; actual print settings.
$_handle_id; The registered event handler id.
$_native-object; The native object provided by the Raku object which registered this event. This a native Gnome::Gtk4::PrintOperation object.
$_widget; The object which registered the signal. User code may have left the object going out of scope.
user
-options; A list of named arguments provided at the.register-signal()
method from Gnome::GObject::Object.